修改以下代码错误:#include <iostream> #include"MyClass.h" using namespace std; class MyClass { public: MyClass() { x = 0; y = 0; } MyClass(int a = 0, int b = 0); private: int x, y; Print(); }; void MyClass::Print() { cout << "x=" << x << endl; cout << "y=" << y << endl; } MyClass::MyClass(int a = 0, int b = 0) { x = a; y = b; } int main() { MyClass obj1, obj2(5, 8); obj1.x = 1; obj1.y = 3; obj1.Print(); obj2.Print(); return 0; }
时间: 2023-12-03 21:02:42 浏览: 70
好的,这段代码中有以下问题:
1. 头文件和类定义放置的顺序不正确,应该先包含头文件再定义类。
2. 类的成员函数 Print() 的声明应该在类的 public 部分,否则无法访问。
3. MyClass 的构造函数声明中,应该移除默认参数值,否则重复定义。
4. Print() 函数定义中,应该在函数名和函数体之间添加返回类型 void。
下面是修改后的代码:
```c++
#include<iostream>
#include"MyClass.h"
using namespace std;
class MyClass {
public:
MyClass(int a = 0, int b = 0);
void Print();
private:
int x, y;
};
MyClass::MyClass(int a, int b) {
x = a;
y = b;
}
void MyClass::Print() {
cout << "x=" << x << endl;
cout << "y=" << y << endl;
}
int main() {
MyClass obj1, obj2(5, 8);
obj1.x = 1;
obj1.y = 3;
obj1.Print();
obj2.Print();
return 0;
}
```
希望这样修改能够帮到您,如有其他问题,欢迎继续询问。
相关问题
修改以下代码中的错误:#include<iostream> #include"MyClass.h" using namespace std; class MyClass { public: MyClass(int a = 0, int b = 0); void Print(); private: int x, y; }; MyClass::MyClass(int a, int b) { x = a; y = b; } void MyClass::Print() { cout << "x=" << x << endl; cout << "y=" << y << endl; } int main() { MyClass obj1, obj2(5, 8); obj1.x = 1; obj1.y = 3; obj1.Print(); obj2.Print(); return 0; }
这段代码没有明显的语法错误,但是存在潜在的逻辑错误。在类的定义中,数据成员 x 和 y 是私有的,不能直接在 main 函数中访问和赋值。应该使用类的公有成员函数来访问和修改这些数据成员。
下面是修改后的代码:
```c++
#include<iostream>
#include"MyClass.h"
using namespace std;
class MyClass {
public:
MyClass(int a = 0, int b = 0);
void Print();
void SetX(int a);
void SetY(int b);
int GetX();
int GetY();
private:
int x, y;
};
MyClass::MyClass(int a, int b) {
x = a;
y = b;
}
void MyClass::Print() {
cout << "x=" << x << endl;
cout << "y=" << y << endl;
}
void MyClass::SetX(int a) {
x = a;
}
void MyClass::SetY(int b) {
y = b;
}
int MyClass::GetX() {
return x;
}
int MyClass::GetY() {
return y;
}
int main() {
MyClass obj1, obj2(5, 8);
obj1.SetX(1);
obj1.SetY(3);
obj1.Print();
obj2.Print();
return 0;
}
```
希望这样修改能够帮到您,如有其他问题,欢迎继续询问。
#include<iostream> using namespace std; private void Fill_array(string, int) {}; int main() { }这段代码 为什么错误
这段 C++ 代码包含了一些常见的语法错误和逻辑问题:
1. **`#include<iostream>`**:这是正确的头文件引入,用于输入输出流操作。
2. **`using namespace std;`**:这是命名空间 `std` 的引入,使得我们能够直接使用 `std` 中的函数、类等。通常在大型项目中需要避免全局命名空间,但在小型示例中可以接受。
3. **`private void Fill_array(string, int) {};`**:这是一个未在任何类中声明的私有成员函数。在 C++ 中,你需要在一个类里面定义方法,并且 `private` 访问修饰符表明该方法只能在类内部访问。
4. **`int main()`**:这是程序的主入口点,但在这个代码片段中它后面缺少了大括号 `{}`,意味着 `main` 函数体的内容没有被包含。
5. **`{}`**:最后一行缺少大括号来包裹 `main` 函数的主体,导致编译器不知道从哪里开始执行代码。
修复这些问题后,你应该像下面这样组织代码:
```cpp
#include <iostream>
class MyClass {
private:
void Fill_array(std::string, int); // 将函数声明移到类里
public:
int main() {
// 在这里添加主函数的具体内容
// 现在函数体应该在大括号内
return 0;
}
};
// 实现函数
void MyClass::Fill_array(std::string s, int value) {
// 函数体...
}
```
阅读全文
相关推荐
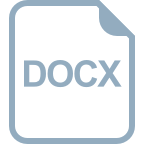
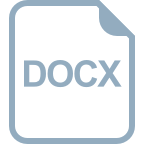
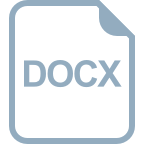












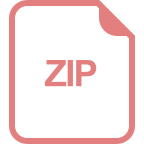
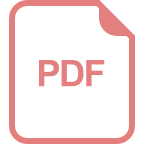