为何import numpy as np from PIL import Image def image_generator(file_paths, batch_size=32): """ 生成器函数,每次返回一个批次的图像数组 """ start_index = 0 end_index = batch_size while start_index < len(file_paths): batch_paths = file_paths[start_index:end_index] images = [] for path in batch_paths: img = Image.open(path) img = img.resize((450, 600)) img = np.asarray(img) images.append(img) images = np.array(images) yield images start_index = end_index end_index += batch_size # 读取所有图像文件路径 file_paths = df['path'].tolist() # 定义空的NumPy数组,用于存储所有图像数据 all_images = np.empty((len(file_paths), 600, 450, 3), dtype=np.uint8) # 使用生成器函数逐批次读取和处理图像,并将每个批次的图像数据存储在上述空数组中 img_gen = image_generator(file_paths) i = 0 for batch_images in img_gen: batch_size = batch_images.shape[0] all_images[i:i+batch_size] = batch_images i += batch_size # 将存储有所有图像数据的NumPy数组赋值给 `df['image']` 列 df['image'] = all_images会出现MemoryError: Unable to allocate 7.48 GiB for an array with shape (9921, 600, 450, 3) and data type uint8报错
时间: 2024-03-26 08:34:50 浏览: 14
这个问题出现的原因是因为在将所有图像数据存储在一个NumPy数组中时,所需的内存超过了系统的可用内存,从而导致内存错误。
解决这个问题的方法是使用生成器函数逐批次读取和处理图像,而不是一次性将所有图像数据存储在一个NumPy数组中。可以在生成器函数中使用`yield`语句逐批次返回图像数据,在每次返回前处理一批图像,这样就可以避免一次性加载所有图像导致内存溢出的问题。
下面是一个示例代码,其中`batch_size`表示每个批次包含的样本数量,`df`是包含图像路径的DataFrame对象:
```
import numpy as np
from PIL import Image
def image_generator(df, batch_size, img_size):
num_samples = len(df)
while True:
for offset in range(0, num_samples, batch_size):
batch_df = df[offset:offset+batch_size]
images = []
for path in batch_df['path']:
img = Image.open(path).resize(img_size)
images.append(np.asarray(img))
X = np.array(images)
yield X
batch_size = 32
img_size = (600, 450)
gen = image_generator(df, batch_size, img_size)
# 读取生成器中的每个批次,并将所有图像数据存储在 `df['image']` 列中
for i, batch_images in enumerate(gen):
start_index = i * batch_size
end_index = start_index + batch_images.shape[0]
df.loc[start_index:end_index, 'image'] = batch_images
```
这样就可以逐批次读取和处理图像,避免一次性加载所有图像导致内存溢出的问题。
相关推荐
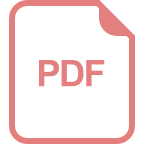
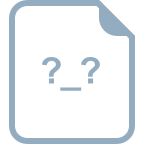
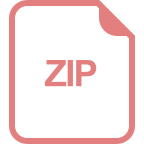














