基于OpenSSL的AES算法
时间: 2023-12-10 18:57:42 浏览: 111
OpenSSL是一个强大的加密库,支持多种加密算法,包括AES算法。以下是使用OpenSSL库实现AES加密和解密的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
#define AES_BLOCK_SIZE 16
// AES加密
int aes_encrypt(const unsigned char *plaintext, int plaintext_len, const unsigned char *key, unsigned char *ciphertext)
{
AES_KEY aes_key;
if (AES_set_encrypt_key(key, 128, &aes_key) < 0)
return -1;
int num_blocks = plaintext_len / AES_BLOCK_SIZE;
int remainder = plaintext_len % AES_BLOCK_SIZE;
for (int i = 0; i < num_blocks; i++)
AES_encrypt(plaintext + i * AES_BLOCK_SIZE, ciphertext + i * AES_BLOCK_SIZE, &aes_key);
if (remainder > 0)
{
unsigned char block[AES_BLOCK_SIZE];
memset(block, 0, AES_BLOCK_SIZE);
memcpy(block, plaintext + num_blocks * AES_BLOCK_SIZE, remainder);
AES_encrypt(block, ciphertext + num_blocks * AES_BLOCK_SIZE, &aes_key);
}
return 0;
}
// AES解密
int aes_decrypt(const unsigned char *ciphertext, int ciphertext_len, const unsigned char *key, unsigned char *plaintext)
{
AES_KEY aes_key;
if (AES_set_decrypt_key(key, 128, &aes_key) < 0)
return -1;
int num_blocks = ciphertext_len / AES_BLOCK_SIZE;
int remainder = ciphertext_len % AES_BLOCK_SIZE;
for (int i = 0; i < num_blocks; i++)
AES_decrypt(ciphertext + i * AES_BLOCK_SIZE, plaintext + i * AES_BLOCK_SIZE, &aes_key);
if (remainder > 0)
{
unsigned char block[AES_BLOCK_SIZE];
memset(block, 0, AES_BLOCK_SIZE);
memcpy(block, ciphertext + num_blocks * AES_BLOCK_SIZE, remainder);
AES_decrypt(block, plaintext + num_blocks * AES_BLOCK_SIZE, &aes_key);
}
return 0;
}
int main()
{
unsigned char key[] = "0123456789ABCDEF";
unsigned char plaintext[] = "Hello, world!";
unsigned char ciphertext[sizeof(plaintext)];
unsigned char decrypted[sizeof(plaintext)];
int plaintext_len = strlen((char*)plaintext);
aes_encrypt(plaintext, plaintext_len, key, ciphertext);
printf("Ciphertext: ");
for (int i = 0; i < plaintext_len; i++)
printf("%02x ", ciphertext[i]);
printf("\n");
aes_decrypt(ciphertext, plaintext_len, key, decrypted);
printf("Decrypted: %s\n", decrypted);
return 0;
}
```
在此例中,我们使用了128位的密钥。如果需要使用256位的密钥,只需将AES_set_encrypt_key和AES_set_decrypt_key的第二个参数改为256即可。
阅读全文
相关推荐
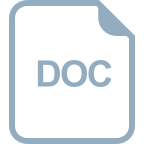
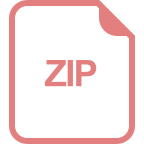
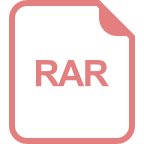
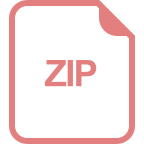
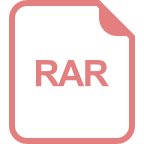
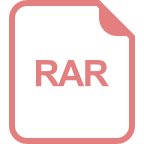
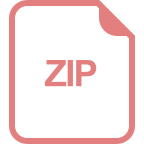
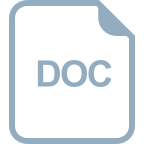







