opencv怎么把图片传递给ffmpeg
时间: 2023-07-05 10:26:19 浏览: 165
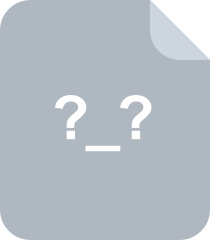
opencv-ffmpeg
要将OpenCV中的图像传递给FFmpeg进行编码或其他处理,可以使用FFmpeg的图像传输协议,该协议允许在不将图像写入磁盘的情况下,将图像传输到FFmpeg进行处理。
以下是一个使用Python和OpenCV将图像传递给FFmpeg的简单示例:
```python
import cv2
import subprocess
width = 640
height = 480
fps = 30
output_url = 'rtmp://your-rtmp-server.com/live/stream-key'
# Open the video capture device
cap = cv2.VideoCapture(0)
cap.set(cv2.CAP_PROP_FRAME_WIDTH, width)
cap.set(cv2.CAP_PROP_FRAME_HEIGHT, height)
cap.set(cv2.CAP_PROP_FPS, fps)
# Start the FFmpeg process with image2pipe input
ffmpeg_cmd = ['ffmpeg', '-f', 'rawvideo', '-pix_fmt', 'bgr24', '-s', f'{width}x{height}', '-r', str(fps), '-i', '-', '-c:v', 'libx264', '-preset', 'veryfast', '-maxrate', '3000k', '-bufsize', '6000k', '-f', 'flv', output_url]
ffmpeg_process = subprocess.Popen(ffmpeg_cmd, stdin=subprocess.PIPE)
# Read frames from the video capture device and send them to FFmpeg
while True:
ret, frame = cap.read()
if not ret:
break
ffmpeg_process.stdin.write(frame.tobytes())
# Close the video capture device and FFmpeg process
cap.release()
ffmpeg_process.stdin.close()
ffmpeg_process.wait()
```
在这个例子中,我们使用OpenCV打开视频捕获设备(通常是摄像头),并将它的输出传递给FFmpeg。我们启动了一个FFmpeg进程,并使用 `-f rawvideo` 告诉FFmpeg输入是原始的视频帧,使用 `-pix_fmt bgr24` 告诉FFmpeg输入像素格式为BGR24,使用 `-s`、`-r` 和 `-i` 分别指定视频帧的分辨率、帧率和输入文件(这里使用 `-i -` 表示从标准输入中读取)。然后,我们使用 `-c:v libx264`、`-preset veryfast`、`-maxrate`、`-bufsize`、`-f` 等选项指定输出编码器和其他编码参数,并使用 `-` 表示输出到标准输出。在Python中,我们可以通过 `stdin` 属性将图像数据写入FFmpeg的标准输入流。最后,我们在循环结束时关闭设备和进程。
阅读全文
相关推荐
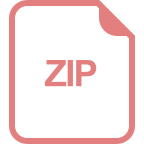

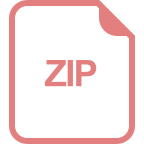
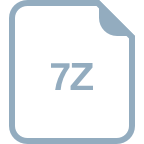
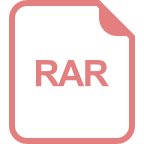
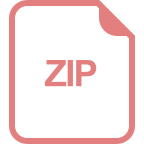
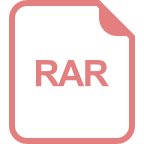
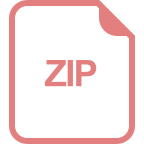
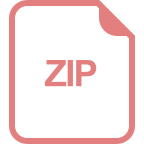
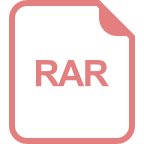
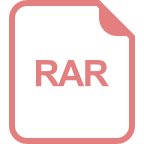





