强化学习中sac算法与q-learning算法的区别和优缺点及其使用条件
时间: 2024-05-23 20:10:04 浏览: 28
SAC算法和Q-learning算法都是强化学习中常用的算法,但它们有着不同的优缺点和使用条件。
首先,Q-learning算法是离散状态空间下的强化学习算法,其优点是能够精确地估计各个状态下每个动作的价值函数,缺点是在连续状态空间下效果不佳,因为需要对状态空间进行离散化处理。此外,Q-learning算法的收敛性并不总是保证。
相比之下,SAC算法是连续状态空间下的强化学习算法,它可以直接对连续状态进行处理,避免了出现的离散化处理问题。SAC算法是一种基于最大熵统计物理学的算法,其优点在于能够处理连续动作空间和不确定性等问题,并且具有更好的收敛性。
使用条件来看,Q-learning算法适用于离散状态空间下,在连续状态空间下会出现精度不足的问题。SAC算法则适用于连续状态空间下,因为其通过直接对连续状态进行处理,可以避免离散化处理的问题。
总之,SAC算法和Q-learning算法各有优缺点,在不同的应用场景下需要选择适合的算法。
相关问题
请给出自动驾驶领域行为决策规划所使用到的经典强化学习算法和最新强化学习算法
在自动驾驶领域的行为决策规划中,经典的强化学习算法和一些最新的强化学习算法都被广泛应用。以下是其中一些常见的算法:
经典强化学习算法:
1. Q-learning:基于价值的强化学习算法,广泛应用于自动驾驶领域的路径规划和行为决策。
2. DQN(Deep Q Network):将深度神经网络与Q-learning相结合,用于处理高维状态和动作空间的问题。
3. A3C(Asynchronous Advantage Actor-Critic):结合了Actor-Critic方法和并行异步训练的算法,用于连续动作空间和大规模问题。
最新强化学习算法:
1. PPO(Proximal Policy Optimization):一种基于策略的强化学习算法,通过近端策略优化来提高样本的利用效率,并且具有较好的收敛性和稳定性。
2. SAC(Soft Actor-Critic):一种基于策略的强化学习算法,通过最大熵原则来平衡探索和利用,适用于连续动作空间和高维状态空间。
3. DDPG(Deep Deterministic Policy Gradient):一种基于策略的强化学习算法,用于处理连续动作空间的问题,并结合了Actor-Critic方法和深度神经网络。
4. MADDPG(Multi-Agent Deep Deterministic Policy Gradient):一种用于多智能体系统中的基于策略的强化学习算法,能够处理多个智能体协同决策的问题。
5. Dreamer:一种基于模型的强化学习算法,结合了模型预测和经验回放的方法,用于处理部分可观测马尔可夫决策过程(POMDP)问题。
需要注意的是,自动驾驶领域的研究和算法发展非常迅速,新的算法不断涌现。以上列举的仅是一部分经典和最新的强化学习算法,而实际应用中可能会结合不同的算法和技术进行系统设计和优化。
请给出强化学习的典型算法和最新算法
强化学习有许多典型算法和最新算法。下面是一些典型的强化学习算法和一些最新的算法:
典型算法:
1. Q-learning:基于价值的强化学习算法,用于解决马尔可夫决策过程(MDP)问题。
2. Sarsa:基于价值的强化学习算法,用于解决MDP问题,并且可以处理带有函数逼近的情况。
3. Policy Gradient:基于策略的强化学习算法,通过直接优化策略函数来学习最优策略。
4. DQN(Deep Q Network):结合了深度神经网络和Q-learning的算法,用于处理高维状态空间的问题。
5. A3C(Asynchronous Advantage Actor-Critic):结合了Actor-Critic方法和并行异步训练的算法,用于处理连续动作空间和大规模问题。
最新算法:
1. SAC(Soft Actor-Critic):一种基于策略的强化学习算法,通过最大熵原则来平衡探索和利用,适用于连续动作空间和高维状态空间。
2. PPO(Proximal Policy Optimization):一种基于策略的强化学习算法,通过近端策略优化来提高样本的利用效率,并且具有较好的收敛性和稳定性。
3. DDPG(Deep Deterministic Policy Gradient):一种基于策略的强化学习算法,用于处理连续动作空间的问题,并结合了Actor-Critic方法和深度神经网络。
4. Rainbow:一种结合了多种强化学习算法的方法,如DQN、Double Q-learning、Prioritized Experience Replay等,用于进一步提升训练稳定性和性能。
5. R2D2(Recurrent Replay Distributed DQN):一种结合了循环神经网络和分布式训练的算法,用于处理部分可观测马尔可夫决策过程(POMDP)问题。
需要注意的是,随着研究的不断进展,新的强化学习算法不断涌现,以上列举的仅是一部分典型算法和最新算法,并不能穷尽所有。研究者们在不同应用领域中也会尝试结合和改进这些算法,以满足具体问题的需求。
相关推荐
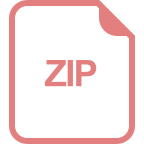
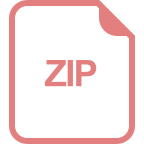
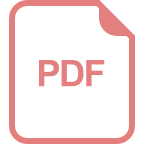












