使用Python计算三维空间中一个点到一个平面的距离的代码
时间: 2023-09-11 15:05:15 浏览: 264
### 回答1:
可以使用以下代码来计算三维空间中一个点到一个平面的距离:
```
import numpy as np
def distance_point_plane(point, plane_normal, plane_point):
d = np.dot(plane_normal, (point - plane_point)) / np.linalg.norm(plane_normal)
return np.abs(d)
point = np.array([1, 2, 3])
plane_normal = np.array([1, 1, 1])
plane_point = np.array([0, 0, 0])
print(distance_point_plane(point, plane_normal, plane_point))
```
在上面的代码中,`point`表示点的坐标,`plane_normal`表示平面的法向量,`plane_point`表示平面上的一个点。
### 回答2:
在Python中,可以通过以下代码来计算三维空间中一个点到一个平面的距离:
```python
import math
def distance_to_plane(point, plane):
# 获取平面上的一点
p0 = plane[0]
# 获取平面的法向量
normal_vector = plane[1]
# 计算点到平面的距离
distance = abs((point[0] - p0[0]) * normal_vector[0] +
(point[1] - p0[1]) * normal_vector[1] +
(point[2] - p0[2]) * normal_vector[2])
distance /= math.sqrt(math.pow(normal_vector[0], 2) +
math.pow(normal_vector[1], 2) +
math.pow(normal_vector[2], 2))
return distance
# 定义点和平面
point = (1, 2, 3)
plane = ((4, 5, 6), (1, 1, 1))
# 计算距离
distance = distance_to_plane(point, plane)
print("点到平面的距离为:", distance)
```
在上述代码中:
- `point` 表示一个三维空间中的点,以元组 `(x, y, z)` 的形式表示。
- `plane` 表示一个三维空间中的平面,以一个包含两个元组的元组 `((p0_x, p0_y, p0_z), (n_x, n_y, n_z))` 的形式表示,其中 `(p0_x, p0_y, p0_z)` 表示平面上的一点,`(n_x, n_y, n_z)` 表示平面的法向量。
- `distance_to_plane` 是一个自定义的函数,它接受一个点和一个平面作为输入,返回点到平面的距离。
- 在函数内部,我们使用点到平面的公式来计算距离,然后将结果返回。
- 最后,我们定义了一个点和一个平面,并通过调用 `distance_to_plane` 函数来计算距离,并打印结果。
### 回答3:
要计算一个三维空间中一个点到一个平面的距离,可以使用以下Python代码:
```python
import math
# 定义平面上的三个点
p1 = [1, 2, 3]
p2 = [4, 5, 6]
p3 = [7, 8, 9]
# 定义待计算距离的点
point = [2, 3, 4]
# 计算平面的法向量
vector1 = [p2[0] - p1[0], p2[1] - p1[1], p2[2] - p1[2]]
vector2 = [p3[0] - p1[0], p3[1] - p1[1], p3[2] - p1[2]]
normal_vector = [vector1[1]*vector2[2] - vector1[2]*vector2[1], vector1[2]*vector2[0] - vector1[0]*vector2[2], vector1[0]*vector2[1] - vector1[1]*vector2[0]]
# 计算点到平面的距离
distance = abs((normal_vector[0]*(point[0] - p1[0])) + (normal_vector[1]*(point[1] - p1[1])) + (normal_vector[2]*(point[2] - p1[2]))) / math.sqrt(normal_vector[0]**2 + normal_vector[1]**2 + normal_vector[2]**2)
print("点到平面的距离为:", distance)
```
以上代码首先定义了平面上的三个点和待计算距离的点。然后计算了平面的法向量,通过法向量公式得到平面方程的法向量。最后通过点到平面的距离公式计算出点到平面的距离,并打印输出结果。
相关推荐
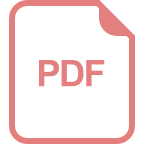
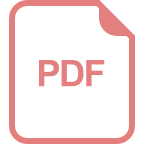
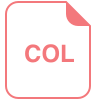










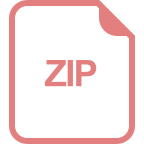
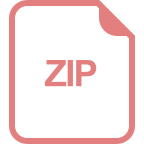