给我打一个神庙逃亡的代码
时间: 2023-12-06 16:45:05 浏览: 81
以下是一个简单的神庙逃亡小游戏的代码,使用 Python 语言编写:
``` python
import random
# 定义迷宫地图
maze = [
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
['#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'],
['#', ' ', '#', ' ', '#', ' ', '#', '#', ' ', '#'],
['#', ' ', '#', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', ' ', '#', '#', '#', '#', ' ', '#', '#', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', ' ', '#', '#', '#', '#', '#', ' ', '#', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#']
]
# 定义初始位置和方向
pos = [1, 1] # 初始位置
dirs = [[0, 1], [1, 0], [0, -1], [-1, 0]] # 可行的方向
dir_names = ['right', 'down', 'left', 'up'] # 方向名称
dir_index = 0 # 当前方向下标
# 开始迷宫逃亡
while True:
# 输出当前位置
print('Current position: ({}, {})'.format(pos[0], pos[1]))
# 检查是否到达终点
if pos == [8, 8]:
print('Congratulations, you escaped from the maze!')
break
# 沿当前方向前进一步
next_pos = [pos[0] + dirs[dir_index][0], pos[1] + dirs[dir_index][1]]
# 判断下一步是否越界或者遇到墙壁
if next_pos[0] < 0 or next_pos[0] > 9 or next_pos[1] < 0 or next_pos[1] > 9 or maze[next_pos[0]][next_pos[1]] == '#':
# 随机选择一个可行的方向
while True:
dir_index = random.randint(0, 3)
next_pos = [pos[0] + dirs[dir_index][0], pos[1] + dirs[dir_index][1]]
if next_pos[0] >= 0 and next_pos[0] <= 9 and next_pos[1] >= 0 and next_pos[1] <= 9 and maze[next_pos[0]][next_pos[1]] != '#':
break
print('Oops, you hit a wall! Turning to {}...'.format(dir_names[dir_index]))
else:
pos = next_pos
```
这个代码实现了一个简单的迷宫逃亡小游戏,其中 `maze` 表示迷宫地图,`pos` 表示当前位置,`dirs` 表示可行的方向,`dir_names` 表示方向名称,`dir_index` 表示当前方向下标。在每一步中,程序会先输出当前位置,并检查是否到达终点。如果没有到达终点,则沿当前方向前进一步,判断下一步是否越界或者遇到墙壁,如果越界或者遇到墙壁,则随机选择一个可行的方向。如果到达终点,则输出恭喜信息,并结束游戏。
相关推荐
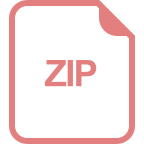














