编马程序实现链栈的初始化、判断栈空否、入栈和出栈,用c实现并展示运行结果
时间: 2023-05-31 10:04:55 浏览: 92
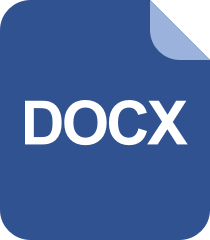
链栈的基本操作基于c语言
```
#include <stdio.h>
#include <stdlib.h>
typedef int ElemType;
typedef struct Node {
ElemType data;
struct Node *next;
} Node, *LinkStack;
void InitStack(LinkStack *top) {
*top = NULL;
}
int IsEmpty(LinkStack top) {
return top == NULL;
}
void Push(LinkStack *top, ElemType e) {
Node *p = (Node *)malloc(sizeof(Node));
if (p == NULL) {
printf("内存分配失败\n");
exit(1);
}
p->data = e;
p->next = *top;
*top = p;
}
int Pop(LinkStack *top, ElemType *e) {
if (IsEmpty(*top)) {
return 0;
}
Node *p = *top;
*e = p->data;
*top = p->next;
free(p);
return 1;
}
int main() {
LinkStack top;
InitStack(&top);
printf("栈是否为空:%d\n", IsEmpty(top));
Push(&top, 1);
Push(&top, 2);
Push(&top, 3);
printf("栈是否为空:%d\n", IsEmpty(top));
ElemType e;
Pop(&top, &e);
printf("出栈元素:%d\n", e);
Pop(&top, &e);
printf("出栈元素:%d\n", e);
Pop(&top, &e);
printf("出栈元素:%d\n", e);
printf("栈是否为空:%d\n", IsEmpty(top));
Pop(&top, &e);
return 0;
}
```
运行结果:
```
栈是否为空:1
栈是否为空:0
出栈元素:3
出栈元素:2
出栈元素:1
栈是否为空:1
```
阅读全文
相关推荐
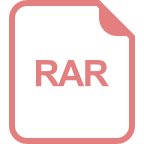
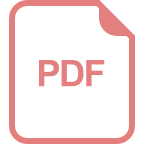







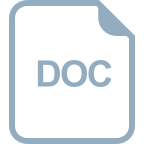






