python 装饰器通用模板
时间: 2023-07-08 07:55:44 浏览: 46
以下是 Python 装饰器的通用模板:
```python
def decorator(func):
def wrapper(*args, **kwargs):
# 在函数调用之前执行的代码
result = func(*args, **kwargs)
# 在函数调用之后执行的代码
return result
return wrapper
```
其中,`decorator` 函数是一个装饰器函数,它接受一个函数作为参数,并返回一个新的函数 `wrapper`。在 `wrapper` 函数内部,可以编写在函数调用前后需要执行的代码。最后,`wrapper` 函数返回原始函数 `func` 的返回值。
使用装饰器时,只需要在需要装饰的函数上方使用 `@decorator` 语法即可。例如:
```python
@decorator
def my_function():
pass
```
这样,`my_function` 函数就被 `decorator` 装饰器修饰了。
相关问题
python 通用的装饰器
Python中的装饰器是一种特殊的函数,用于修改其他函数的功能。装饰器可以在不修改原函数代码的情况下,为函数添加额外的功能或者修改函数的行为。下面是几种常见的通用装饰器:
1. 函数装饰器:函数装饰器是最常见的装饰器类型,它可以在函数定义之前使用@符号来修饰函数。函数装饰器接受一个函数作为参数,并返回一个新的函数,通常用于在原函数执行前后添加额外的逻辑。例如:
```python
def decorator(func):
def wrapper(*args, **kwargs):
# 添加额外的逻辑
result = func(*args, **kwargs)
# 添加额外的逻辑
return result
return wrapper
@decorator
def my_function():
# 函数的原始逻辑
pass
```
2. 类装饰器:类装饰器是一种使用类来装饰函数或方法的装饰器。类装饰器通过实现`__call__`方法来实现装饰器的功能。例如:
```python
class Decorator:
def __init__(self, func):
self.func = func
def __call__(self, *args, **kwargs):
# 添加额外的逻辑
result = self.func(*args, **kwargs)
# 添加额外的逻辑
return result
@Decorator
def my_function():
# 函数的原始逻辑
pass
```
3. 带参数的装饰器:装饰器也可以接受参数,可以通过在装饰器外再包裹一层函数来实现。例如:
```python
def decorator_with_args(arg1, arg2):
def decorator(func):
def wrapper(*args, **kwargs):
# 使用传入的参数arg1和arg2
# 添加额外的逻辑
result = func(*args, **kwargs)
# 添加额外的逻辑
return result
return wrapper
return decorator
@decorator_with_args(arg1, arg2)
def my_function():
# 函数的原始逻辑
pass
```
python web前端通用模板
对于 Python 的 Web 前端开发,有很多通用模板可供选择。以下是其中一些常用的 Python Web 前端通用模板:
1. Flask:Flask 是一个轻量级的 Python Web 框架,提供了简洁而灵活的方式来构建 Web 应用程序。它不提供专门的前端模板,但可以与常见的前端框架(如 Bootstrap、Jinja2 等)进行集成,实现前后端分离。
2. Django:Django 是一个功能强大且全面的 Python Web 框架,提供了许多内置的功能和工具。它使用 Django 模板语言(Django Templating Language)作为默认的前端模板引擎,可以方便地进行模板渲染和数据传递。
3. Pyramid:Pyramid 是一个灵活且可扩展的 Python Web 框架,它支持多种前端模板引擎,如 Chameleon、Jinja2、Mako 等。开发者可以根据自己的需求选择适合的模板引擎进行前端开发。
4. Bottle:Bottle 是一个轻量级的 Python Web 框架,它提供了简单而直观的 API,适合快速构建小型的 Web 应用程序。虽然 Bottle 自身没有默认的前端模板引擎,但可以与其他第三方模板引擎(如 Jinja2、Mako 等)进行集成。
这些通用模板可以帮助你快速搭建 Web 应用程序的前端部分,并且提供了丰富的功能和工具来简化开发过程。根据个人的喜好和项目需求,你可以选择适合自己的模板来进行前端开发。
相关推荐
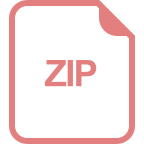
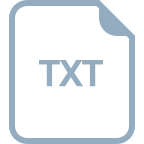
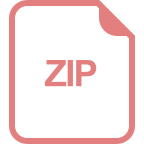












