c语言代码用顺序表求两集合并集
时间: 2024-04-30 14:24:22 浏览: 57
以下是使用顺序表实现求两个集合的并集的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int length;
} SqList;
void InitList(SqList *L) {
L->length = 0;
}
void InsertList(SqList *L, int e) {
if (L->length == MAX_SIZE) {
printf("List is full.\n");
return;
}
L->data[L->length] = e;
L->length++;
}
void MergeList(SqList *L1, SqList L2) {
int i, j, k;
for (i = 0; i < L2.length; i++) {
for (j = 0; j < L1->length; j++) {
if (L2.data[i] == L1->data[j]) {
break;
}
}
if (j == L1->length) {
InsertList(L1, L2.data[i]);
}
}
}
int main() {
SqList L1, L2;
int n, m, i, e;
printf("Enter the number of elements in set 1: ");
scanf("%d", &n);
printf("Enter the elements of set 1: ");
for (i = 0; i < n; i++) {
scanf("%d", &e);
InsertList(&L1, e);
}
printf("Enter the number of elements in set 2: ");
scanf("%d", &m);
printf("Enter the elements of set 2: ");
for (i = 0; i < m; i++) {
scanf("%d", &e);
InsertList(&L2, e);
}
MergeList(&L1, L2);
printf("The union of set 1 and set 2 is: ");
for (i = 0; i < L1.length; i++) {
printf("%d ", L1.data[i]);
}
printf("\n");
return 0;
}
```
其中,`SqList` 是一个结构体类型,用于表示顺序表。`InitList` 函数用于初始化一个顺序表,`InsertList` 函数用于向顺序表中插入元素,`MergeList` 函数用于求两个集合的并集。在 `main` 函数中,首先输入两个集合的元素,然后调用 `MergeList` 函数求并集,最后输出结果。
阅读全文
相关推荐
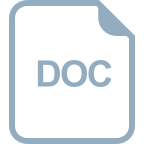
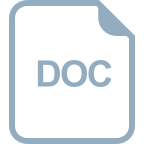
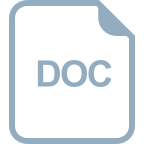














