from PIL import Image import numpy as np import matplotlib.pyplot as plt img = Image.open("work/Lena_RGB.jpg") plt.imshow(img) plt.show() plt.close() img_arr = np.array(img) h,w = img_arr.shape gray_levels = np.arange(256) freq = np.zeros(256) for i in range(h): for j in range(w): freq[img_arr[i, j]] += 1 prob = freq / (h * w) 检查并改正代码
时间: 2023-12-24 07:02:49 浏览: 32
在代码中有一个缺失的部分,需要计算出图像的灰度值后再计算灰度直方图。
以下是更正后的代码:
```python
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
img = Image.open("work/Lena_RGB.jpg")
plt.imshow(img)
plt.show()
plt.close()
img_gray = img.convert('L') # 转换为灰度图像
img_arr = np.array(img_gray)
h, w = img_arr.shape
gray_levels = np.arange(256)
freq = np.zeros(256)
for i in range(h):
for j in range(w):
freq[img_arr[i, j]] += 1
prob = freq / (h * w)
plt.bar(gray_levels, prob)
plt.show()
```
在这个代码中,我们首先使用 `convert()` 函数将 RGB 彩色图像转换为灰度图像。然后,我们计算出图像的灰度值后再计算灰度直方图。最后,我们使用 `bar()` 函数绘制灰度直方图。
相关问题
用python自己编写代码实现AHE算法并用plt输出
好的,AHE算法是一种自适应直方图均衡化的算法,可以增强图像的局部对比度。以下是一个用Python实现AHE算法的示例代码,并使用matplotlib绘制输出结果。
```python
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
def AHE(img, clipLimit):
img = np.array(img)
height, width = img.shape
hist, bins = np.histogram(img.flatten(), 256, [0, 256])
# 计算累积分布函数
cdf = hist.cumsum()
cdf = (cdf / float(cdf[-1])) * 255
# 计算裁剪限制
clipLimit = clipLimit * (height * width) / 256
# 对像素值进行裁剪
for i in range(256):
if cdf[i] > clipLimit:
cdf[i] = clipLimit
# 重新计算累积分布函数
cdf = cdf.cumsum()
cdf = (cdf / float(cdf[-1])) * 255
# 映射像素值
img_eq = np.interp(img.flatten(), bins[:-1], cdf).astype(np.uint8)
img_eq = np.reshape(img_eq, img.shape)
return img_eq
# 读取图像
img = Image.open('lena.png').convert('L')
# 应用AHE算法
img_eq = AHE(img, clipLimit=4.0)
# 显示原始图像和均衡化后的图像
fig, axs = plt.subplots(1, 2, figsize=(12, 6))
axs[0].imshow(img, cmap='gray')
axs[0].set_title('Original')
axs[1].imshow(img_eq, cmap='gray')
axs[1].set_title('AHE')
plt.show()
```
在上面的代码中,我们使用PIL库读取图像,并使用AHE函数应用AHE算法。我们使用matplotlib库绘制原始图像和均衡化后的图像。
请注意,上述代码中的clipLimit参数控制裁剪限制。这是一个阈值,用于控制像素值的裁剪。较高的clipLimit值会导致更强的削弱效果,可能会导致图像的过度增强。
用matplotlib重新写上述的程序,16位二进制数据本身就是RGB565格式,无需再次转换。
好的,以下是用matplotlib重新写的程序:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取二进制文件
with open('lena_256x256_rgb565.bin', 'rb') as f:
data = f.read()
# 将二进制数据转换成numpy数组
arr = np.frombuffer(data, dtype=np.uint16)
# 将RGB565格式的数据拆分成R、G、B三个通道
r = ((arr & 0xf800) >> 11) << 3
g = ((arr & 0x07e0) >> 5) << 2
b = (arr & 0x001f) << 3
# 将R、G、B三个通道合并成RGB图像
rgb = np.dstack((r, g, b))
# 显示图像
plt.imshow(rgb)
plt.show()
```
这个程序与之前的程序相比,最大的不同在于使用了matplotlib库来显示图像。我们可以使用`plt.imshow`函数来显示numpy数组对应的图像,而无需使用PIL库。同时,由于16位二进制数据本身就是RGB565格式,因此无需再次转换。
相关推荐
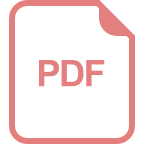
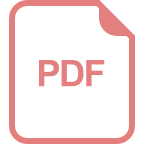











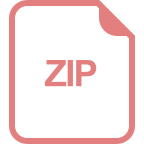
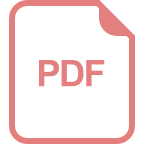