怎么使用这个函数初始化串口3HAL_StatusTypeDef HAL_UART_Init(UART_HandleTypeDef huart) { / Check the UART handle allocation / if (huart == NULL) { return HAL_ERROR; } / Check the parameters / if (huart->Init.HwFlowCtl != UART_HWCONTROL_NONE) { / The hardware flow control is available only for USART1, USART2, USART3 and USART6. Except for STM32F446xx devices, that is available for USART1, USART2, USART3, USART6, UART4 and UART5. / assert_param(IS_UART_HWFLOW_INSTANCE(huart->Instance)); assert_param(IS_UART_HARDWARE_FLOW_CONTROL(huart->Init.HwFlowCtl)); } else { assert_param(IS_UART_INSTANCE(huart->Instance)); } assert_param(IS_UART_WORD_LENGTH(huart->Init.WordLength)); assert_param(IS_UART_OVERSAMPLING(huart->Init.OverSampling)); if (huart->gState == HAL_UART_STATE_RESET) { / Allocate lock resource and initialize it / huart->Lock = HAL_UNLOCKED; #if (USE_HAL_UART_REGISTER_CALLBACKS == 1) UART_InitCallbacksToDefault(huart); if (huart->MspInitCallback == NULL) { huart->MspInitCallback = HAL_UART_MspInit; } / Init the low level hardware / huart->MspInitCallback(huart); #else / Init the low level hardware : GPIO, CLOCK / HAL_UART_MspInit(huart); #endif / (USE_HAL_UART_REGISTER_CALLBACKS) / } huart->gState = HAL_UART_STATE_BUSY; / Disable the peripheral / __HAL_UART_DISABLE(huart); / Set the UART Communication parameters / UART_SetConfig(huart); / In asynchronous mode, the following bits must be kept cleared: - LINEN and CLKEN bits in the USART_CR2 register, - SCEN, HDSEL and IREN bits in the USART_CR3 register./ CLEAR_BIT(huart->Instance->CR2, (USART_CR2_LINEN | USART_CR2_CLKEN)); CLEAR_BIT(huart->Instance->CR3, (USART_CR3_SCEN | USART_CR3_HDSEL | USART_CR3_IREN)); / Enable the peripheral / __HAL_UART_ENABLE(huart); / Initialize the UART state */ huart->ErrorCode = HAL_UART_ERROR_NONE; huart->gState = HAL_UART_STATE_READY; huart->RxState = HAL_UART_STATE_READY; return HAL_OK; }
时间: 2023-12-03 20:45:35 浏览: 193
这个函数是用来初始化UART串口的,具体的使用方法如下:
1. 首先需要定义一个`UART_HandleTypeDef`类型的结构体变量`huart`,并对其中的各个成员变量进行配置,例如:
```
UART_HandleTypeDef huart;
huart.Instance = USART3;
huart.Init.BaudRate = 115200;
huart.Init.WordLength = UART_WORDLENGTH_8B;
huart.Init.StopBits = UART_STOPBITS_1;
huart.Init.Parity = UART_PARITY_NONE;
huart.Init.Mode = UART_MODE_TX_RX;
huart.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart.Init.OverSampling = UART_OVERSAMPLING_16;
```
2. 调用`HAL_UART_Init()`函数进行初始化,将上述结构体变量传入该函数即可。
```
HAL_StatusTypeDef result = HAL_UART_Init(&huart);
if (result != HAL_OK) {
// 初始化失败,进行错误处理
} else {
// 初始化成功,进行后续操作
}
```
在该函数内部,会先进行一些参数检查,然后根据参数配置寄存器,最终完成UART串口的初始化。
相关问题
这个函数怎么使用HAL_StatusTypeDef HAL_UART_Init(UART_HandleTypeDef *huart) { /* Check the UART handle allocation */ if (huart == NULL) { return HAL_ERROR; } /* Check the parameters */ if (huart->Init.HwFlowCtl != UART_HWCONTROL_NONE) { /* The hardware flow control is available only for USART1, USART2, USART3 and USART6. Except for STM32F446xx devices, that is available for USART1, USART2, USART3, USART6, UART4 and UART5. */ assert_param(IS_UART_HWFLOW_INSTANCE(huart->Instance)); assert_param(IS_UART_HARDWARE_FLOW_CONTROL(huart->Init.HwFlowCtl)); } else { assert_param(IS_UART_INSTANCE(huart->Instance)); } assert_param(IS_UART_WORD_LENGTH(huart->Init.WordLength)); assert_param(IS_UART_OVERSAMPLING(huart->Init.OverSampling)); if (huart->gState == HAL_UART_STATE_RESET) { /* Allocate lock resource and initialize it */ huart->Lock = HAL_UNLOCKED; #if (USE_HAL_UART_REGISTER_CALLBACKS == 1) UART_InitCallbacksToDefault(huart); if (huart->MspInitCallback == NULL) { huart->MspInitCallback = HAL_UART_MspInit; } /* Init the low level hardware */ huart->MspInitCallback(huart); #else /* Init the low level hardware : GPIO, CLOCK */ HAL_UART_MspInit(huart); #endif /* (USE_HAL_UART_REGISTER_CALLBACKS) */ } huart->gState = HAL_UART_STATE_BUSY; /* Disable the peripheral */ __HAL_UART_DISABLE(huart); /* Set the UART Communication parameters */ UART_SetConfig(huart); /* In asynchronous mode, the following bits must be kept cleared: - LINEN and CLKEN bits in the USART_CR2 register, - SCEN, HDSEL and IREN bits in the USART_CR3 register.*/ CLEAR_BIT(huart->Instance->CR2, (USART_CR2_LINEN | USART_CR2_CLKEN)); CLEAR_BIT(huart->Instance->CR3, (USART_CR3_SCEN | USART_CR3_HDSEL | USART_CR3_IREN)); /* Enable the peripheral */ __HAL_UART_ENABLE(huart); /* Initialize the UART state */ huart->ErrorCode = HAL_UART_ERROR_NONE; huart->gState = HAL_UART_STATE_READY; huart->RxState = HAL_UART_STATE_READY; return HAL_OK; }
这个函数是HAL库(STM32的驱动库)中的UART初始化函数,用于初始化指定的UART外设。它的参数是一个UART_HandleTypeDef类型的指针,其中包含了UART的初始化参数和外设的实例。函数执行过程中会进行一系列的参数检查,包括检查参数是否为空、硬件流控制是否可用、数据位长度是否合法等等。如果UART的gState状态为HAL_UART_STATE_RESET,那么它会先初始化底层硬件资源(包括GPIO和时钟),否则会直接进行配置和使能。最后,函数会将UART的状态设置为HAL_UART_STATE_READY,表示初始化完成。
HAL_StatusTypeDef HAL_UART_Init(UART_HandleTypeDef *huart) { /* Check the UART handle allocation */ if (huart == NULL) { return HAL_ERROR; } /* Check the parameters */ if (huart->Init.HwFlowCtl != UART_HWCONTROL_NONE) { /* The hardware flow control is available only for USART1, USART2, USART3 and USART6. Except for STM32F446xx devices, that is available for USART1, USART2, USART3, USART6, UART4 and UART5. */ assert_param(IS_UART_HWFLOW_INSTANCE(huart->Instance)); assert_param(IS_UART_HARDWARE_FLOW_CONTROL(huart->Init.HwFlowCtl)); } else { assert_param(IS_UART_INSTANCE(huart->Instance)); } assert_param(IS_UART_WORD_LENGTH(huart->Init.WordLength)); assert_param(IS_UART_OVERSAMPLING(huart->Init.OverSampling)); if (huart->gState == HAL_UART_STATE_RESET) { /* Allocate lock resource and initialize it */ huart->Lock = HAL_UNLOCKED; #if (USE_HAL_UART_REGISTER_CALLBACKS == 1) UART_InitCallbacksToDefault(huart); if (huart->MspInitCallback == NULL) { huart->MspInitCallback = HAL_UART_MspInit; } /* Init the low level hardware */ huart->MspInitCallback(huart); #else /* Init the low level hardware : GPIO, CLOCK */ HAL_UART_MspInit(huart); #endif /* (USE_HAL_UART_REGISTER_CALLBACKS) */ } huart->gState = HAL_UART_STATE_BUSY; /* Disable the peripheral */ __HAL_UART_DISABLE(huart); /* Set the UART Communication parameters */ UART_SetConfig(huart); /* In asynchronous mode, the following bits must be kept cleared: - LINEN and CLKEN bits in the USART_CR2 register, - SCEN, HDSEL and IREN bits in the USART_CR3 register.*/ CLEAR_BIT(huart->Instance->CR2, (USART_CR2_LINEN | USART_CR2_CLKEN)); CLEAR_BIT(huart->Instance->CR3, (USART_CR3_SCEN | USART_CR3_HDSEL | USART_CR3_IREN)); /* Enable the peripheral */ __HAL_UART_ENABLE(huart); /* Initialize the UART state */ huart->ErrorCode = HAL_UART_ERROR_NONE; huart->gState = HAL_UART_STATE_READY; huart->RxState = HAL_UART_STATE_READY; return HAL_OK; }
这是HAL库中初始化UART外设的函数。它会首先检查传入的参数是否合法,然后根据传入的参数设置UART通信参数,最后使能UART外设并将状态设置为READY。在这个函数中还会调用HAL_UART_MspInit()或者用户自定义的MspInitCallback()函数来初始化外设的引脚和时钟等低级硬件。
阅读全文
相关推荐
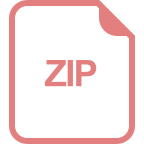
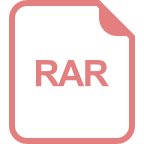














