给我用c++写一个银行排队系统的程序
时间: 2023-09-11 16:05:16 浏览: 139
### 回答1:
#include <stdio.h>int main()
{
int number_of_people = 0; // 人数
int i; printf("请输入排队的人数:");
scanf("%d", &number_of_people); printf("开始排队:\n");
for(i=1; i<=number_of_people; i++)
{
printf("%d号顾客\n", i);
} printf("排队结束!\n"); return 0;
}
### 回答2:
以下是使用C语言编写的一个简单的银行排队系统的程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义队列结构
typedef struct Node {
int data;
struct Node* next;
} QueueNode;
typedef struct {
QueueNode* front;
QueueNode* rear;
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = NULL;
}
// 入队
void enqueue(Queue* q, int data) {
QueueNode* newNode = (QueueNode*)malloc(sizeof(QueueNode));
newNode->data = data;
newNode->next = NULL;
if (q->rear == NULL) {
q->front = q->rear = newNode;
} else {
q->rear->next = newNode;
q->rear = newNode;
}
}
// 出队
int dequeue(Queue* q) {
if (q->front == NULL) {
printf("队列为空\n");
return -1;
}
QueueNode* temp = q->front;
int data = temp->data;
q->front = q->front->next;
if (q->front == NULL) {
q->rear = NULL;
}
free(temp);
return data;
}
// 打印队列中的元素
void printQueue(Queue q) {
QueueNode* current = q.front;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Queue q;
initQueue(&q);
printf("欢迎使用银行排队系统!\n");
while (1) {
int choice, customerNumber;
printf("请选择操作:\n");
printf("1. 客户取号\n");
printf("2. 客户办理业务\n");
printf("3. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入客户号码:");
scanf("%d", &customerNumber);
enqueue(&q, customerNumber);
printf("客户 %d 号已取号\n", customerNumber);
break;
case 2:
if (q.front == NULL) {
printf("当前没有客户在排队\n");
} else {
int servedCustomer = dequeue(&q);
printf("正在为客户 %d 号办理业务\n", servedCustomer);
printf("当前队列:");
printQueue(q);
}
break;
case 3:
printf("感谢使用银行排队系统!\n");
exit(0);
default:
printf("输入无效,请重新选择\n");
break;
}
}
return 0;
}
```
这个程序实现了一个简单的银行排队系统,具有以下功能:
1. 客户可以取号来排队。
2. 客户可以办理业务,会依次处理队列中的客户。
3. 可以退出系统。
主要的数据结构是一个队列,使用链表实现。程序使用循环来提供连续的服务。可以根据需要进行扩展,例如加入客户姓名等信息。
### 回答3:
银行排队系统是一个常见的应用程序,可以使用C语言编写。以下是一个简单的示例代码,演示了银行排队系统的基本功能。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10
typedef struct {
int queue[MAX_QUEUE_SIZE];
int front;
int rear;
} Queue;
void initQueue(Queue *q) {
q->front = 0;
q->rear = 0;
}
int isQueueEmpty(Queue *q) {
return (q->front == q->rear);
}
int isQueueFull(Queue *q) {
return (q->rear == MAX_QUEUE_SIZE);
}
void enqueue(Queue *q, int data) {
if (isQueueFull(q)) {
printf("Queue is full. Cannot enqueue more data.\n");
return;
}
q->queue[q->rear++] = data;
}
int dequeue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue is empty. Cannot dequeue data.\n");
return -1;
}
return q->queue[q->front++];
}
void displayQueue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue is empty.\n");
return;
}
printf("Queue: ");
for (int i = q->front; i < q->rear; i++) {
printf("%d ", q->queue[i]);
}
printf("\n");
}
int main() {
Queue bankQueue;
initQueue(&bankQueue);
// 模拟客户排队
enqueue(&bankQueue, 1);
enqueue(&bankQueue, 2);
enqueue(&bankQueue, 3);
// 展示当前排队顺序
displayQueue(&bankQueue);
// 第一个客户离开
dequeue(&bankQueue);
// 展示更新后的排队顺序
displayQueue(&bankQueue);
return 0;
}
```
以上程序中,银行排队系统使用队列数据结构实现。程序初始化一个存储整数的队列,并提供了一些基本操作函数如初始化队列、判空、判满、入队、出队和展示队列等。使用`enqueue`函数将客户加入队列,使用`dequeue`函数将第一个客户移出队列,并使用`displayQueue`函数展示当前的排队顺序。
这只是一个简单的示例,实际应用中可能需要更复杂的逻辑和功能,比如顾客的优先级调整、多线程处理等。但以上代码提供了一个基本的框架,可以根据需求进行扩展和修改。
阅读全文
相关推荐


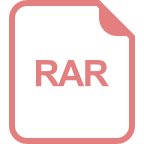



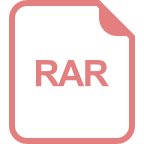
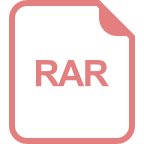






