编写程序使得admin⻆色的用户能访问index.html和admin.html这两个页面,而user用户只能访 问index.html这个页面
时间: 2023-12-14 15:39:05 浏览: 136
这个问题涉及到Web应用程序的权限控制。一种简单的实现方法是在Web服务器上进行配置,比如使用Apache的.htaccess文件来控制访问权限。
针对这个问题,可以在Web服务器上创建一个.htaccess文件,并在其中设置适当的规则来限制访问。例如:
```
# 允许admin用户访问index.html和admin.html页面
AuthType Basic
AuthName "Restricted Content"
AuthUserFile /path/to/.htpasswd
Require user admin
<FilesMatch "(index|admin)\.html$">
Require valid-user
</FilesMatch>
# 允许所有用户访问index.html页面
<FilesMatch "index\.html$">
Allow from all
Satisfy any
</FilesMatch>
```
上述配置中,首先使用Basic认证方式对访问进行身份验证,要求用户输入用户名和密码。然后,只允许admin用户访问index.html和admin.html页面,其他用户无法访问。最后,允许所有用户访问index.html页面,不需要身份验证。
需要注意的是,这种方式只是一种简单的实现方法,安全性可能不够高,还需要根据具体情况进行调整和完善。
相关问题
编写程序使用isUserInRole()使得admin⻆色的用户能访问index.html和admin.html这两个页面,而user用户只能访 问index.html这个页面
这个问题涉及到Web应用程序的安全性,需要使用Web容器提供的安全机制来实现。
以下是一个在Java Web应用程序中使用Servlet和JSP实现上述要求的示例代码:
在web.xml配置文件中添加以下内容:
```xml
<security-role>
<role-name>admin</role-name>
</security-role>
<security-constraint>
<web-resource-collection>
<web-resource-name>Admin Pages</web-resource-name>
<url-pattern>/admin.html</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>admin</role-name>
</auth-constraint>
</security-constraint>
<security-constraint>
<web-resource-collection>
<web-resource-name>Public Pages</web-resource-name>
<url-pattern>/index.html</url-pattern>
</web-resource-collection>
</security-constraint>
```
在Servlet或JSP页面中使用以下代码来检查用户角色并决定是否允许访问:
```java
if (request.isUserInRole("admin")) {
// 允许访问admin.html页面
} else {
// 只允许访问index.html页面
}
```
请注意,上述代码仅为示例代码,具体实现取决于您使用的Web容器和编程语言。
使用isUserInRole()编写代码实现admin⻆色的用户能访问index.html和admin.html这两个页面,而user用户只能访 问index.html这个页面
下面是一个使用`isUserInRole()`方法实现访问控制的示例代码:
```java
@WebServlet("/index.html")
public class IndexServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
if (request.isUserInRole("admin")) {
// admin用户可以访问index.html和admin.html
request.getRequestDispatcher("admin.html").forward(request, response);
} else if (request.isUserInRole("user")) {
// user用户只能访问index.html
request.getRequestDispatcher("index.html").forward(request, response);
} else {
// 未登录的用户重定向到登录页面
response.sendRedirect("login.html");
}
}
}
@WebServlet("/admin.html")
public class AdminServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
if (request.isUserInRole("admin")) {
// admin用户可以访问index.html和admin.html
request.getRequestDispatcher("admin.html").forward(request, response);
} else {
// 非admin用户重定向到index.html
response.sendRedirect("index.html");
}
}
}
```
在上面的代码中,`doGet()`方法首先使用`isUserInRole()`方法检查用户的角色,然后根据用户角色的不同,来决定是否允许访问对应的页面。如果用户没有登录,那么就会重定向到登录页面。
阅读全文
相关推荐
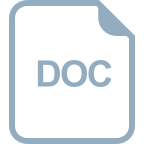
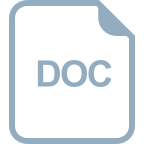
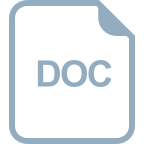
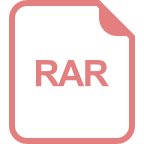
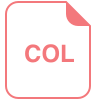
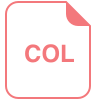
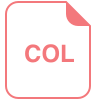
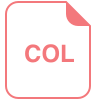
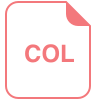
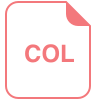
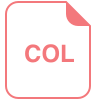
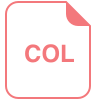
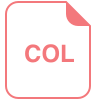
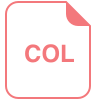
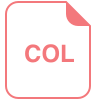
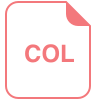
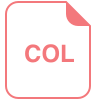