删除链表中的相同元素代码思路
时间: 2023-06-03 19:03:45 浏览: 66
欢迎使用 ChitGPT 进行对话,我会尽力回答您的问题。关于您提出的问题,我可以为您提供一份删除链表中相同元素的代码,具体思路如下:
1. 首先定义一个指针 p1 指向链表的头节点,定义一个指针 p2 指向链表的第二个节点,p3 指向 p2 的前一个节点。
2. 对于每个 p2 节点,遍历整个链表,查找是否有和它值相同的节点。
3. 如果找到了相同值的节点,就把 p2 从链表中删除,并释放其占用的内存。
4. 如果没有找到相同值的节点,就把 p2 指针向后移动一位。
5. 如果 p2 指向链表的尾节点,那么结束遍历。
实现这个删除链表中相同元素的功能,我们可以写出以下代码:
#include <stdio.h>
#include <stdlib.h>
struct Node {
int val;
struct Node *next;
};
struct Node *createList(int arr[], int n) {
struct Node *head = NULL, *tail = NULL;
for (int i = 0; i < n; i++) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->val = arr[i];
node->next = NULL;
if (tail == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
void deleteDuplicates(struct Node *head) {
struct Node *p1 = head, *p2 = head->next, *p3 = head;
while (p2 != NULL) {
struct Node *p = head;
int found = 0;
while (p != p2) {
if (p->val == p2->val) {
found = 1;
break;
}
p = p->next;
}
if (found) {
p3->next = p2->next;
free(p2);
p2 = p3->next;
} else {
p3 = p2;
p2 = p2->next;
}
}
}
void printList(struct Node *head) {
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 2, 4, 5, 6, 1};
int n = sizeof(arr) / sizeof(arr[0]);
struct Node *head = createList(arr, n);
deleteDuplicates(head);
printList(head);
return 0;
}
这样,我们就实现了删除链表中相同元素的功能。如果您还有其他问题需要帮助解答,可以随时向我提出。
相关推荐
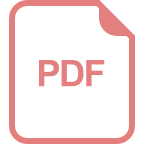









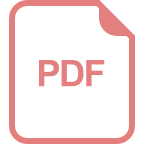
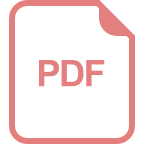