Java后端飞机大战绘制初始界面显示背景界面,界面向下移动,到临界位置重置的讲解
时间: 2023-08-21 11:01:50 浏览: 135
好的,让我更详细地解释一下之前给您的答案。
在Java中,您可以使用Swing或JavaFX等图形界面库来创建GUI应用程序。在这个问题中,我们将使用Swing来创建一个JPanel来承载背景图像,并使用Timer定期更新背景的位置。
首先,我们需要创建一个JPanel来承载背景图像。我们可以创建一个名为BackgroundScroll的类,并让它继承JPanel类。在这个类的构造函数中,我们设置JPanel的首选大小,并使用ImageIcon加载背景图像。在这里,我们将使用名为background.png的图像文件作为背景图像。您需要将其替换为您想要使用的图像文件的名称。
```
public class BackgroundScroll extends JPanel {
private static final int PANEL_WIDTH = 800;
private static final int PANEL_HEIGHT = 600;
private Image background;
public BackgroundScroll() {
setPreferredSize(new Dimension(PANEL_WIDTH, PANEL_HEIGHT));
background = new ImageIcon("background.png").getImage();
}
}
```
接下来,我们需要在JPanel中绘制背景图像。我们可以在BackgroundScroll类中覆盖paintComponent方法,并使用Graphics对象在其上绘制图像。在这里,我们绘制了两个背景图像,一个在另一个之上,以使背景图像在滚动时无缝连接。
```
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(background, 0, backgroundPosition, null);
g.drawImage(background, 0, backgroundPosition + BACKGROUND_HEIGHT, null);
}
```
现在,我们需要使用Timer定期更新背景的位置。我们可以在BackgroundScroll类中实现ActionListener接口,并覆盖actionPerformed方法。在这个方法中,我们增加背景的位置,并检查它是否到达了临界位置。如果是,我们将背景位置重置为0。
```
public class BackgroundScroll extends JPanel implements ActionListener {
private static final int BACKGROUND_MOVE_SPEED = 5;
private static final int BACKGROUND_HEIGHT = 800;
private static final int RESET_POSITION = -BACKGROUND_HEIGHT;
private int backgroundPosition;
public BackgroundScroll() {
//...
Timer timer = new Timer(50, this);
timer.start();
}
//...
public void actionPerformed(ActionEvent e) {
backgroundPosition += BACKGROUND_MOVE_SPEED;
if (backgroundPosition >= RESET_POSITION) {
backgroundPosition = 0;
}
repaint();
}
}
```
最后,我们需要将BackgroundScroll JPanel添加到JFrame中,并显示它。我们可以在main方法中创建一个JFrame,并将BackgroundScroll JPanel添加到它的内容面板中。
```
public static void main(String[] args) {
JFrame frame = new JFrame("Background Scroll");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new BackgroundScroll());
frame.pack();
frame.setVisible(true);
}
```
这样,我们就实现了Java后端飞机大战绘制初始界面显示背景界面,界面向下移动,到临界位置重置的功能。
希望这个解释能够帮助您更好地理解之前给您的答案。
阅读全文
相关推荐
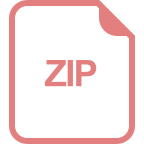






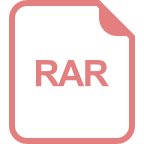
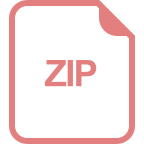
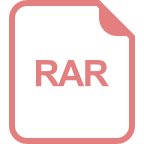
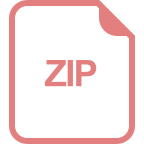
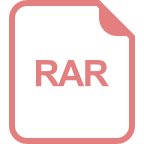
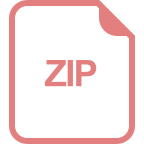
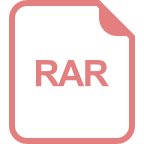
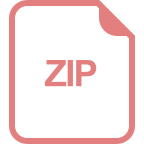