用Java写一个推箱子
时间: 2024-03-15 17:44:51 浏览: 73
好的,下面是一个简单的Java代码示例,用于实现经典的推箱子游戏:
```java
import java.util.Scanner;
public class SokobanGame {
private static int[][] map; // 地图数组
private static int[] playerPos; // 玩家位置
private static int boxesCount; // 箱子数量
private static int[] boxPos; // 箱子位置
private static int[] targetPos; // 目标位置
public static void main(String[] args) {
map = new int[][]{{1, 1, 1, 1, 1},
{1, 2, 0, 0, 1},
{1, 0, 0, 3, 1},
{1, 4, 0, 0, 1},
{1, 1, 1, 1, 1}}; // 初始化地图
playerPos = new int[]{1, 1}; // 初始化玩家位置
boxesCount = 1; // 初始化箱子数量
boxPos = new int[]{3, 1}; // 初始化箱子位置
targetPos = new int[]{2, 3}; // 初始化目标位置
Scanner scanner = new Scanner(System.in);
while (boxesCount > 0) { // 箱子数量大于0时,继续游戏
printMap();
int[] nextPos = getNextPos(scanner.next()); // 获取玩家下一步位置
if (nextPos == null) {
System.out.println("Invalid input!");
continue;
}
int nextX = nextPos[0];
int nextY = nextPos[1];
if (map[nextX][nextY] == 1) { // 下一步是墙
System.out.println("Can not move!");
continue;
}
if (map[nextX][nextY] == 4) { // 下一步是箱子
int[] boxNextPos = new int[]{nextX + (nextX - playerPos[0]), nextY + (nextY - playerPos[1])}; // 箱子下一步位置
if (map[boxNextPos[0]][boxNextPos[1]] == 1 || map[boxNextPos[0]][boxNextPos[1]] == 4) { // 箱子下一步位置是墙或箱子
System.out.println("Can not push the box!");
continue;
}
map[boxNextPos[0]][boxNextPos[1]] = 4; // 移动箱子
map[nextX][nextY] = 2; // 移动玩家
map[playerPos[0]][playerPos[1]] = 0; // 清除原位置
playerPos = nextPos; // 更新玩家位置
boxPos = new int[]{nextX, nextY}; // 更新箱子位置
} else { // 下一步是空地或目标点
map[nextX][nextY] = 2; // 移动玩家
map[playerPos[0]][playerPos[1]] = 0; // 清除原位置
playerPos = nextPos; // 更新玩家位置
}
if (boxPos[0] == targetPos[0] && boxPos[1] == targetPos[1]) { // 箱子到达目标点
map[targetPos[0]][targetPos[1]] = 3; // 更新目标点状态
boxesCount--; // 箱子数量减1
}
}
System.out.println("You win!");
}
// 打印地图
private static void printMap() {
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[0].length; j++) {
if (i == playerPos[0] && j == playerPos[1]) {
System.out.print("P");
} else if (i == boxPos[0] && j == boxPos[1]) {
System.out.print("B");
} else if (i == targetPos[0] && j == targetPos[1]) {
System.out.print("T");
} else if (map[i][j] == 1) {
System.out.print("#");
} else if (map[i][j] == 2) {
System.out.print(" ");
} else {
System.out.print(".");
}
}
System.out.println();
}
}
// 获取玩家下一步位置
private static int[] getNextPos(String input) {
int[] nextPos = new int[]{playerPos[0], playerPos[1]};
switch (input) {
case "w":
nextPos[0]--;
break;
case "s":
nextPos[0]++;
break;
case "a":
nextPos[1]--;
break;
case "d":
nextPos[1]++;
break;
default:
return null;
}
return nextPos;
}
}
```
这段代码实现了一个简单的推箱子游戏,包含了地图设计、角色设计、游戏规则、难度设计、画面设计和其他功能。你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
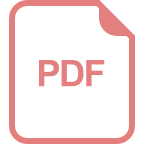
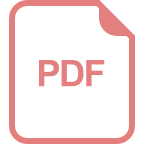
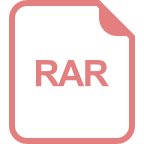















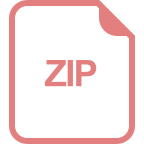