用Java写一个简单的推箱子游戏
时间: 2023-09-04 16:11:42 浏览: 77
推箱子游戏是一种经典的益智游戏,现在我们可以用Java来实现它。以下是一个简单的推箱子游戏的示例代码:
```java
import java.util.Scanner;
public class PushBoxGame {
private int row; // 地图行数
private int col; // 地图列数
private int playerRow; // 玩家所在行
private int playerCol; // 玩家所在列
private int[][] map; // 地图
public PushBoxGame() {
// 初始化地图
row = 5;
col = 8;
playerRow = 2;
playerCol = 2;
map = new int[][]{
{1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 0, 0, 0, 0, 1},
{1, 0, 2, 0, 3, 0, 0, 1},
{1, 0, 0, 0, 1, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1, 1}
};
}
public void start() {
Scanner scanner = new Scanner(System.in);
while (true) {
printMap();
System.out.println("请输入方向(w、a、s、d):");
String direction = scanner.nextLine();
if (direction.equals("w")) {
move(-1, 0);
} else if (direction.equals("a")) {
move(0, -1);
} else if (direction.equals("s")) {
move(1, 0);
} else if (direction.equals("d")) {
move(0, 1);
}
if (isWin()) {
System.out.println("恭喜你,游戏胜利!");
break;
}
}
}
private void printMap() {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (i == playerRow && j == playerCol) {
System.out.print("P ");
} else if (map[i][j] == 1) {
System.out.print("# ");
} else if (map[i][j] == 2) {
System.out.print("B ");
} else if (map[i][j] == 3) {
System.out.print("G ");
} else {
System.out.print(". ");
}
}
System.out.println();
}
}
private void move(int rowOffset, int colOffset) {
int newRow = playerRow + rowOffset;
int newCol = playerCol + colOffset;
if (map[newRow][newCol] == 0) {
// 空格子,直接移动
playerRow = newRow;
playerCol = newCol;
} else if (map[newRow][newCol] == 2) {
// 推箱子
int boxNewRow = newRow + rowOffset;
int boxNewCol = newCol + colOffset;
if (map[boxNewRow][boxNewCol] == 0) {
// 空格子,移动箱子和玩家
map[newRow][newCol] = 0;
map[boxNewRow][boxNewCol] = 2;
playerRow = newRow;
playerCol = newCol;
}
}
}
private boolean isWin() {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (map[i][j] == 3) {
return false;
}
}
}
return true;
}
}
```
在这个示例代码中,我们使用了一个二维整数数组来表示地图,其中1表示墙,0表示空格,2表示箱子,3表示目标位置。玩家所在的位置用playerRow和playerCol来表示。在start方法中,我们循环接收用户输入的方向,并调用move方法来移动玩家和箱子。isWin方法用来判断是否已经达成胜利条件。最后,在main方法中创建PushBoxGame对象并调用start方法即可开始游戏。
阅读全文
相关推荐
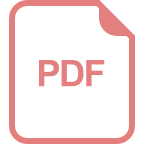
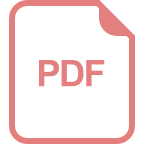
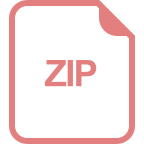















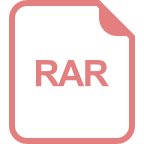