用 java 写一个推箱子代码
时间: 2023-05-15 13:08:11 浏览: 77
好的,以下是一个简单的 Java 推箱子游戏的代码:
```
import java.util.Scanner;
public class Sokoban {
private static int playerRow;
private static int playerCol;
private static char[][] board = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'}
};
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
initializeBoard();
printBoard();
while (true) {
System.out.print("Enter direction (w/a/s/d): ");
char direction = scanner.next().charAt(0);
movePlayer(direction);
printBoard();
if (isGameWon()) {
System.out.println("Congratulations, you won!");
break;
}
}
}
private static void initializeBoard() {
playerRow = 1;
playerCol = 1;
board[4][4] = '$';
board[5][5] = '@';
board[6][6] = '*';
}
private static void printBoard() {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
private static void movePlayer(char direction) {
int newRow = playerRow;
int newCol = playerCol;
switch (direction) {
case 'w':
newRow--;
break;
case 'a':
newCol--;
break;
case 's':
newRow++;
break;
case 'd':
newCol++;
break;
}
if (board[newRow][newCol] == ' ') {
board[playerRow][playerCol] = ' ';
playerRow = newRow;
playerCol = newCol;
board[playerRow][playerCol] = '@';
} else if (board[newRow][newCol] == '$') {
int boxNewRow = newRow;
int boxNewCol = newCol;
switch (direction) {
case 'w':
boxNewRow--;
break;
case 'a':
boxNewCol--;
break;
case 's':
boxNewRow++;
break;
case 'd':
boxNewCol++;
break;
}
if (board[boxNewRow][boxNewCol] == ' ') {
board[playerRow][playerCol] = ' ';
playerRow = newRow;
playerCol = newCol;
board[playerRow][playerCol] = '@';
board[boxNewRow][boxNewCol] = '$';
}
}
}
private static boolean isGameWon() {
return board[6][6] == '$' && board[7][7] == '@' && board[8][8] == '*';
}
}
```
这是一个简单的控制台推箱子游戏,使用了二维字符数组来表示游戏地图,其中 '#' 表示墙,' ' 表示空地,'@' 表示玩家,'$' 表示箱子,'*' 表示目标位置。玩家可以通过输入 w/a/s/d 来移动,如果玩家移动到了箱子旁边,可以将箱子推动到相邻的空地上。当所有的箱子都被推到了目标位置上时,游戏胜利。
阅读全文
相关推荐
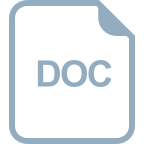
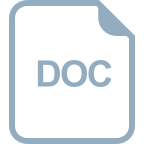
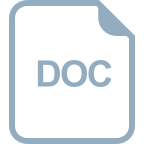





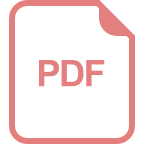




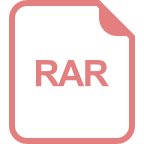
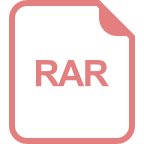



