tflite文件是什么数组
时间: 2024-06-07 16:07:28 浏览: 21
tflite文件是TensorFlow Lite模型的压缩文件格式,其中包含了模型的权重参数和计算图结构等信息。在tflite文件中,模型的参数和结构被编码成一个或多个二进制数组,其中每个数组对应模型中的一个张量。这些数组可以被加载到内存中,以便在移动设备或嵌入式设备上进行推理。tflite文件的使用可以帮助我们在资源有限的设备上实现高效的机器学习推理。
相关问题
tflite文件是什么
TFLite是TensorFlow Lite的简称,是一种用于在移动设备和嵌入式设备上运行机器学习模型的轻量级格式和运行时引擎。TFLite文件(.tflite)是指经过优化和转换后的机器学习模型文件,它可以在移动设备上进行高效的推理。
TFLite文件是通过对TensorFlow模型进行转换和量化而生成的。在转换过程中,模型被转换为一组操作序列,这些操作可以在移动设备上高效地执行。此外,TFLite还使用量化技术来减小模型的大小,从而在资源受限的设备上提供更快的推理速度和更小的存储空间占用。
TFLite文件可以在移动应用程序中使用TFLite运行时引擎加载和运行。它提供了一组API来加载模型并进行推理,使开发者能够在移动设备上轻松地集成机器学习功能。
总结起来,TFLite文件是经过优化和转换后的机器学习模型文件,用于在移动设备和嵌入式设备上进行高效的推理。
tflite模型是什么
TFLite (TensorFlow Lite) 是 TensorFlow 的移动和嵌入式设备部署解决方案之一。TFLite 模型是 TensorFlow 模型经过优化和转换后的轻量级模型格式,它旨在在资源受限的设备上实现高效的推理。TFLite 模型通常具有较小的文件大小和较低的计算复杂度,适用于移动设备、嵌入式设备和物联网设备等场景。
TFLite 模型可以使用 TensorFlow 进行训练,并通过 TensorFlow 转换工具将其转换为 TFLite 格式。这种转换过程会剔除一些不必要的操作、减少精度等,以适应资源受限的设备。然后,可以使用 TFLite 解释器加载和运行 TFLite 模型,实现在设备上进行推理任务。
总结起来,TFLite 模型是经过优化和转换的 TensorFlow 模型,适用于移动和嵌入式设备上进行高效的推理任务。
相关推荐
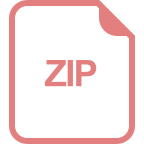
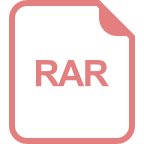












