handler.obtainmessage
时间: 2023-04-29 13:02:55 浏览: 57
handler.obtainMessage是一个Android中的方法,用于获取一个Message对象,以便在Handler中进行处理。它可以用来发送消息,更新UI等操作。在使用时,需要传入一个消息类型和消息内容,然后将其发送到Handler中进行处理。
相关问题
class ImageDownloader (private val url: String, private val imageView: ImageView, private val progressBar: ProgressBar) : Handler.Callback { private val handler = Handler(Looper.getMainLooper(), this) override fun handleMessage(msg: Message): Boolean { if (msg.what == 0) { imageView.setImageBitmap(msg.obj as Bitmap) progressBar.visibility = View.GONE } else if (msg.what == 1) { progressBar.progress = msg.arg1 } return true } fun download() { Thread { val bitmap = downloadImageFromUrl(url) handler.sendMessage(handler.obtainMessage(0, bitmap)) }.start() } private fun downloadImageFromUrl(url: String): Bitmap? { var bitmap: Bitmap? = null try { val urlConnection = URL(url).openConnection() urlConnection.connect() val inputStream = urlConnection.inputStream val totalSize = urlConnection.contentLength val data = ByteArray(1024) var downloadedSize = 0 var bufferLength: Int while (inputStream.read(data).also { bufferLength = it } != -1) { downloadedSize += bufferLength val progress = (downloadedSize * 100 / totalSize) handler.sendMessage(handler.obtainMessage(1, progress, 0)) bitmap = BitmapFactory.decodeByteArray(data, 0, bufferLength) } inputStream.close() } catch (e: Exception) { Log.e("ImageDownloader", "Error downloading image", e) } return bitmap } } 报出Process: com.example.imagedownloader, PID: 4842 java.lang.NullPointerException: null cannot be cast to non-null type android.graphics.Bitmap错误 修改代码
在 `downloadImageFromUrl` 函数中,应该将 `BitmapFactory.decodeByteArray` 转换成可空类型,以避免在无法解码字节数组时抛出 `NullPointerException`。修改后的代码如下:
```
private fun downloadImageFromUrl(url: String): Bitmap? {
var bitmap: Bitmap? = null
try {
val urlConnection = URL(url).openConnection()
urlConnection.connect()
val inputStream = urlConnection.inputStream
val totalSize = urlConnection.contentLength
val data = ByteArray(1024)
var downloadedSize = 0
var bufferLength: Int
while (inputStream.read(data).also { bufferLength = it } != -1) {
downloadedSize += bufferLength
val progress = (downloadedSize * 100 / totalSize)
handler.sendMessage(handler.obtainMessage(1, progress, 0))
bitmap = BitmapFactory.decodeByteArray(data, 0, bufferLength)?.let { it } // 加上安全转换
}
inputStream.close()
} catch (e: Exception) {
Log.e("ImageDownloader", "Error downloading image", e)
}
return bitmap
}
```
public class MusicService extends Service { private MediaPlayer player; private Timer timer; public MusicService() {} @Override public IBinder onBind(Intent intent){ return new MusicControl(); } @Override public void onCreate(){ super.onCreate(); player=new MediaPlayer();//创建音乐播放器对象 } public void addTimer(){ //添加计时器用于设置音乐播放器中的播放进度条 if(timer==null){ timer=new Timer();//创建计时器对象 TimerTask task=new TimerTask() { @Override public void run() { if (player==null) return; int duration=player.getDuration();//获取歌曲总时长 int currentPosition=player.getCurrentPosition();//获取播放进度 Message msg=Music_Activity.handler.obtainMessage();//创建消息对象 //将音乐的总时长和播放进度封装至消息对象中 //Bundl 相当于一个包 用来存放duration和 currentPosition并封装起来发送到MusicActivity中 Bundle bundle=new Bundle(); bundle.putInt("duration",duration); bundle.putInt("currentPosition",currentPosition); msg.setData(bundle); //将消息发送到主线程的消息队列 Music_Activity.handler.sendMessage(msg); } }; //开始计时任务后的5毫秒,第一次执行task任务,以后每500毫秒执行一次 timer.schedule(task,5,500); } }代码解释
这段代码是一个Android应用中的音乐播放服务,用于播放音乐并向其他组件(如活动)提供音乐播放相关的功能。具体来说,它包含一个MediaPlayer对象用于实现音乐播放功能。它还包含一个计时器,用于定期读取MediaPlayer对象的播放进度并将其发送给其他组件。在音乐播放服务被绑定时,它会返回一个MusicControl对象,用于控制音乐播放。在音乐播放服务被创建时,它会创建一个MediaPlayer对象。在addTimer()函数中,它会创建一个计时器对象,并将一个TimerTask对象添加到计时器中。该TimerTask对象的run()方法会读取MediaPlayer对象的播放进度,并将其封装到一个消息对象中发送给其他组件。注意,该代码中的Music_Activity.handler应该是在其他地方定义并初始化过的。
相关推荐
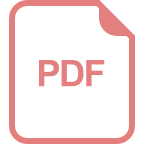
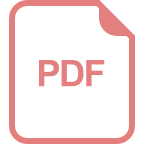












