对原始图像进行以下形式的几何变换:缩放,旋转,平移,裁剪,镜像变换并放在同一个窗口的六个子窗口内的Python代码
时间: 2024-02-21 14:56:38 浏览: 22
这里给出使用Python的OpenCV库实现这一过程的代码:
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('original_image.jpg')
# 缩放变换
scale_percent = 50 # 设置缩放比例
width = int(img.shape[1] * scale_percent / 100)
height = int(img.shape[0] * scale_percent / 100)
dim = (width, height)
resized_img = cv2.resize(img, dim, interpolation=cv2.INTER_AREA)
# 旋转变换
angle = 45 # 设置旋转角度
(rows, cols) = resized_img.shape[:2] # 获取图像的行和列
M = cv2.getRotationMatrix2D((cols / 2, rows / 2), angle, 1) # 构建旋转矩阵
rotated_img = cv2.warpAffine(resized_img, M, (cols, rows))
# 平移变换
tx, ty = 100, 50 # 设置平移量
M = np.float32([[1, 0, tx], [0, 1, ty]]) # 构建平移矩阵
translated_img = cv2.warpAffine(rotated_img, M, (cols, rows))
# 裁剪变换
x, y, w, h = 50, 50, 200, 200 # 设置裁剪区域的坐标和大小
cropped_img = translated_img[y:y+h, x:x+w]
# 镜像变换
mirror_img = cv2.flip(cropped_img, 1) # 1表示沿y轴镜像,0表示沿x轴镜像
# 将变换后的图像放在同一个窗口的六个子窗口内
cv2.imshow('Original Image', img)
cv2.imshow('Resized Image', resized_img)
cv2.imshow('Rotated Image', rotated_img)
cv2.imshow('Translated Image', translated_img)
cv2.imshow('Cropped Image', cropped_img)
cv2.imshow('Mirrored Image', mirror_img)
cv2.waitKey(0) # 等待用户按下任意按键
cv2.destroyAllWindows() # 关闭所有窗口
```
运行以上代码,即可在同一个窗口的六个子窗口内看到原始图像经过缩放、旋转、平移、裁剪、镜像变换后的效果。
相关推荐
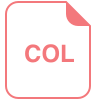
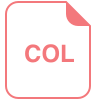
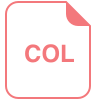
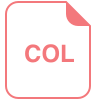













