c++ 按时间排序 目录下文件数 删除旧的文件 保持一定数量文件
时间: 2024-11-15 08:29:57 浏览: 17
在 C++ 中,如果你想按时间顺序对目录下的文件进行排序,并删除旧文件以保持特定数量,可以使用 `std::filesystem` 库(C++17 及以上版本引入),它提供了一套操作文件系统功能的现代工具。以下是简要步骤:
1. 包含头文件并获取目录内容:
```cpp
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
```
2. 获取指定目录下的文件列表,并按时间戳排序:
```cpp
fs::directory_iterator start_dir("/path/to/directory");
fs::directory_iterator end_dir;
std::vector<fs::file_info> files;
for (auto it = start_dir; it != end_dir; ++it) {
if (it->is_regular_file()) { // 只处理普通文件
files.push_back(*it);
}
}
// 对文件信息按照时间戳排序,例如:
bool compare_time(const fs::file_info& a, const fs::file_info& b) {
return a.last_write_time() < b.last_write_time();
}
std::sort(files.begin(), files.end(), compare_time);
```
3. 删除过期文件(假设你想保留前5个最新的文件):
```cpp
int keep_files = 5;
if (files.size() > keep_files) {
for (size_t i = keep_files; i < files.size(); ++i) {
fs::remove(files[i].path()); // 删除多余的文件
}
}
```
注意:上述代码仅用于教学目的,实际应用时要考虑错误处理和资源管理。
阅读全文
相关推荐
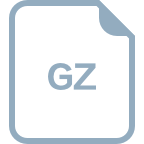


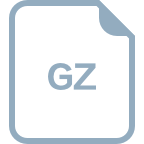



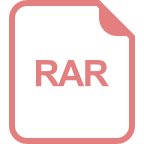









