动态链表存贮数据长整数、高精度运算c语言代码
时间: 2023-10-10 08:11:15 浏览: 109
以下是动态链表存储数据长整数、高精度运算的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 判断链表是否为空
int isListEmpty(struct ListNode *head) {
return head == NULL;
}
// 在链表尾部插入节点
void insertNode(struct ListNode **head, int val) {
struct ListNode *newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct ListNode *cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
// 从字符串转换为链表
struct ListNode* listFromString(char *str) {
int len = strlen(str);
struct ListNode *head = NULL;
for (int i = len - 1; i >= 0; i--) {
int val = str[i] - '0';
insertNode(&head, val);
}
return head;
}
// 从链表转换为字符串
char* stringFromList(struct ListNode *head) {
char *str = (char*)malloc(sizeof(char) * 10000);
int index = 0;
while (head != NULL) {
str[index++] = head->val + '0';
head = head->next;
}
str[index] = '\0';
return str;
}
// 高精度加法
struct ListNode* add(struct ListNode* l1, struct ListNode* l2) {
struct ListNode *result = NULL;
int carry = 0;
while (l1 != NULL || l2 != NULL || carry != 0) {
int sum = carry;
if (l1 != NULL) {
sum += l1->val;
l1 = l1->next;
}
if (l2 != NULL) {
sum += l2->val;
l2 = l2->next;
}
carry = sum / 10;
sum %= 10;
insertNode(&result, sum);
}
return result;
}
// 高精度减法
struct ListNode* subtract(struct ListNode* l1, struct ListNode* l2) {
struct ListNode *result = NULL;
int borrow = 0;
while (l1 != NULL || l2 != NULL) {
int sub = borrow;
if (l1 != NULL) {
sub += l1->val;
l1 = l1->next;
}
if (l2 != NULL) {
sub -= l2->val;
l2 = l2->next;
}
if (sub < 0) {
sub += 10;
borrow = -1;
}
else {
borrow = 0;
}
insertNode(&result, sub);
}
while (!isListEmpty(result) && result->val == 0) {
struct ListNode *temp = result;
result = result->next;
free(temp);
}
return result;
}
// 高精度乘法
struct ListNode* multiply(struct ListNode* l1, struct ListNode* l2) {
struct ListNode *result = NULL;
struct ListNode *cur1 = l1;
while (cur1 != NULL) {
struct ListNode *cur2 = l2;
struct ListNode *temp = NULL;
int carry = 0;
while (cur2 != NULL || carry != 0) {
int mul = carry;
if (cur2 != NULL) {
mul += cur1->val * cur2->val;
cur2 = cur2->next;
}
carry = mul / 10;
mul %= 10;
insertNode(&temp, mul);
}
int zeros = 0;
while (zeros--) {
insertNode(&temp, 0);
}
result = add(result, temp);
cur1 = cur1->next;
}
return result;
}
// 高精度除法
struct ListNode* divide(struct ListNode* l1, struct ListNode* l2) {
struct ListNode *result = NULL;
struct ListNode *cur1 = l1;
while (cur1 != NULL) {
struct ListNode *temp = NULL;
int quotient = 0;
while (1) {
temp = multiply(l2, listFromString("0"));
for (int i = 0; i < quotient; i++) {
insertNode(&temp, 0);
}
if (cur1->val < stringFromList(temp)[0] - '0') {
break;
}
quotient++;
}
result = multiply(result, listFromString("10"));
insertNode(&result, quotient);
cur1 = cur1->next;
}
return result;
}
int main() {
char *str1 = "123456789";
char *str2 = "987654321";
struct ListNode *l1 = listFromString(str1);
struct ListNode *l2 = listFromString(str2);
struct ListNode *sum = add(l1, l2);
struct ListNode *diff = subtract(l1, l2);
struct ListNode *product = multiply(l1, l2);
struct ListNode *quotient = divide(l1, l2);
printf("Sum: %s\n", stringFromList(sum));
printf("Difference: %s\n", stringFromList(diff));
printf("Product: %s\n", stringFromList(product));
printf("Quotient: %s\n", stringFromList(quotient));
return 0;
}
```
阅读全文
相关推荐
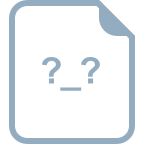
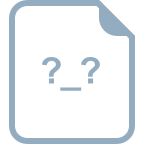
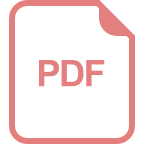
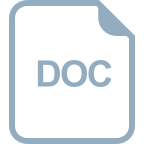
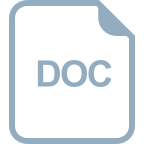


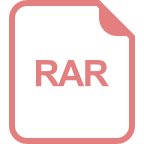
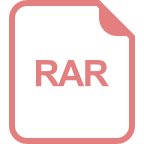
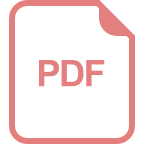
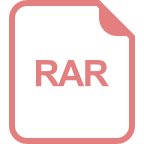
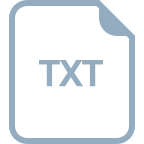
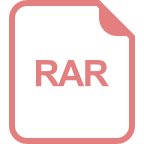
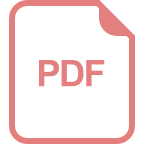
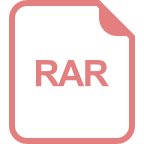
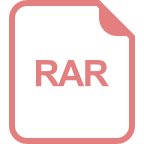
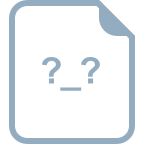
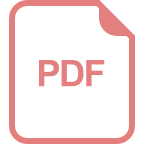