t=np.arange(0.0,2.0,0.1) s=np.sin(t*np.pi)什么意思
时间: 2024-06-07 12:10:52 浏览: 15
这是一个 Python 代码片段,其中:
- `np.arange(0.0, 2.0, 0.1)` 生成了一个数组,从 0.0 开始,以步长 0.1,一直到 2.0,不包含 2.0。
- `t` 是这个数组的名称,可以用于后续的操作。
- `np.pi` 是一个常量,代表圆周率 π。
- `t * np.pi` 将数组 `t` 中的每个元素都乘以 π。
- `np.sin()` 是一个函数,它将传入的数组中的每个元素都计算其正弦值。
- `s` 是保存计算结果的数组名称。
综合起来,这段代码生成了一个从 0 到 2(不包含 2)的数组 `t`,并计算了该数组中每个元素的正弦值,将结果保存在数组 `s` 中。
相关问题
解读:#11_5用折线图绘制正弦和余弦曲线,以及其它显示汉字方法。 import numpy as np import matplotlib . pyplot as plt import matplotlib . fontmanagerasm #使用关键参数 fname 导入字体文件(华文楷体) myfont = fm . FontProperties ( fname = r ' C :\ Windows \ Fonts \ STKAITI . ttf ') x = np . arange (0.0,2.0* np . pi +0.01,0.01) yl = np . sin ( x ) y2= np . cos ( x ) plt . plot ( x , yl , label ='正弦') plt . plot ( x ,y2, label ='余弦') #在0-360度范围,每隔30度设置1个刻度;设置相应的字符串刻度 xt = np . arange (0.0,2.0* np . pi + np . pi /12, np . pi /6) xts =['(}度'. format ( i ) for i in range (0,390,30)] # fontproperties 表示所用字体, rotation 表示刻度标识旋转角度(45度) plt . xticks ( xt , xts , fontproperties - myfont , rotation =45) plt . xlabel (' x ﹣变量', fontproperties = myfont , fontsize =18) plt . ylabel (' y ﹣正弦余弦函数值', fontproperties =' simsun ', fontsize =18)#宋体 plt . title (' sin - cos 函数图像', fontproperties =' STLITI ', fontsize =24)#隶书 plt . legend ( prop - myfont ) #图例选择字体用 prop 关键字 plt . gridO plt . tight _ layout ) plt . show (0 #自变量取值要密,才能成曲线#计算正弦函数值 #紧凑布局,能完整显示图形
这段代码是用于绘制正弦和余弦函数的折线图。首先,通过导入 numpy 和 pyplot 模块,并使用 fontmanager 模块中的 FontProperties 类导入字体文件(华文楷体),定义了 x 的取值范围,并计算出 yl 和 y2 的值。接着,通过 plot 函数将 x 和 yl、y2 绘制成折线图,并设置了标签 label 和图例 legend。然后,通过 arange 函数设置 x 轴的刻度范围和相应的字符串刻度,并使用 format 函数生成刻度字符串。接下来,使用 xticks 函数将刻度值和字符串刻度代替数值刻度,并设置刻度字体和旋转角度。通过 xlabel 和 ylabel 函数设置 x 轴和 y 轴的标签和字体大小,通过 title 函数设置标题和字体大小。最后,通过 legend 函数选择字体,并通过 grid 函数添加网格线。tight_layout 函数可以使图形布局更加紧凑,使得图形能够完整显示。图例选择字体用 prop 关键字。
import matplotlib as mpl import matplotlib.pyplot as plt plt.subplots_adjust(left=None, bottom=None, right=None, top=None, wspace=None, hspace=0.5) t=np.arange(0.0,2.0,0.1) s=np.sin(t*np.pi) plt.subplot(2,2,1) #要生成两行两列,这是第一个图 import numpy as np import matplotlib.pyplot as plt x = np.arange(1,13) y1 = np.array([53673, 57571, 58905, 55239, 49661, 49510, 49163, 57311, 59187, 60074, 57109, 52885]) plt.plot(x, y1) plt.title('近13天登录人数') plt.show() plt.subplot(2,2,2) #两行两列,这是第二个图 import numpy as np import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) x = np.arange(12) y = np.array([70887, 64957, 62508, 66471, 54972, 46245, 64083, 67090, 64991, 88504, 79404, 68253,]) bar_width = 0.3 plt.bar(x, y, tick_label=['1月', '2月', '3月', '4月', '5月', '6月', '7月', "8月", "9月", "10月", '11月', '12月'],width=bar_width) plt.title('近12个月人数') plt.show() plt.subplot(2,2,3)#两行两列,这是第三个图 import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) plt.style.use('fivethirtyeight') languages = ['steam', 'ubisoft'] popularity = [78,22] plt.pie(popularity) plt.tight_layout() plt.pie(popularity, labels=languages, autopct='%1.1f%%') plt.title('游戏平台登录占比') plt.subplot(2,2,4)#两行两列,这是第四个图 import numpy as np from matplotlib import pyplot as plt ages_x = [1, 2, 3, 4, 5, ] x_indexes = np.arange(len(ages_x)) width = 0.33 dev_y = [64050, 65168, 75588, 75590, 60097] py_dev_y = [57809, 56101, 70605, 63437, 56756] plt.bar(x_indexes, dev_y, width=width, label="2022") plt.bar(x_indexes + width, py_dev_y, width=width, label="2023") plt.xlabel("月份") plt.ylabel("平均在线人数") plt.title("2022和2023一到五月在线人数对比") plt.legend() plt.xticks(ticks = x_indexes, labels = ages_x) plt.show()
你刚才的代码中,第三个子图中的饼图有一些问题。虽然你使用了pie函数绘制了饼图,但是没有正确地添加标签和百分比。正确的代码应该是这样的:
```python
plt.subplot(2,2,3)
plt.subplots_adjust(top=0.85)
plt.style.use('fivethirtyeight')
languages = ['steam', 'ubisoft']
popularity = [78,22]
plt.pie(popularity, labels=languages, autopct='%1.1f%%')
plt.title('游戏平台登录占比')
plt.show()
```
这样做可以让饼图正确地显示游戏平台的登录占比,并显示出每个部分所占的百分比。
相关推荐
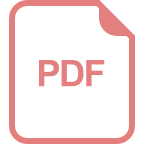
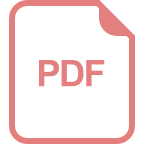












