请用C++写出二叉排序树的查找、插入、建立、删除的动态查找表和打印算法。
时间: 2023-08-08 21:12:37 浏览: 94
二叉排序树是一种动态查找表,支持查找、插入、删除等操作。下面是用C++实现二叉排序树的查找、插入、建立、删除以及打印算法的示例代码:
```c++
#include <iostream>
using namespace std;
// 二叉排序树的结构体
struct BSTNode {
int data;
BSTNode* left;
BSTNode* right;
};
// 查找操作
BSTNode* search(BSTNode* root, int key) {
if (root == NULL || root->data == key) {
return root;
}
if (key < root->data) {
return search(root->left, key);
} else {
return search(root->right, key);
}
}
// 插入操作
BSTNode* insert(BSTNode* root, int key) {
if (root == NULL) {
root = new BSTNode;
root->data = key;
root->left = root->right = NULL;
} else if (key < root->data) {
root->left = insert(root->left, key);
} else {
root->right = insert(root->right, key);
}
return root;
}
// 建立二叉排序树
BSTNode* buildBST(int* arr, int n) {
BSTNode* root = NULL;
for (int i = 0; i < n; i++) {
root = insert(root, arr[i]);
}
return root;
}
// 查找最小值
BSTNode* findMin(BSTNode* root) {
if (root == NULL) {
return NULL;
}
while (root->left != NULL) {
root = root->left;
}
return root;
}
// 删除操作
BSTNode* remove(BSTNode* root, int key) {
if (root == NULL) {
return NULL;
}
if (key < root->data) {
root->left = remove(root->left, key);
} else if (key > root->data) {
root->right = remove(root->right, key);
} else {
if (root->left == NULL) {
BSTNode* temp = root->right;
delete root;
return temp;
} else if (root->right == NULL) {
BSTNode* temp = root->left;
delete root;
return temp;
} else {
BSTNode* temp = findMin(root->right);
root->data = temp->data;
root->right = remove(root->right, temp->data);
}
}
return root;
}
// 中序遍历打印
void inorder(BSTNode* root) {
if (root != NULL) {
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
}
int main() {
int arr[] = {5, 3, 7, 1, 9, 6, 4, 2, 8};
int n = sizeof(arr) / sizeof(arr[0]);
// 建立二叉排序树
BSTNode* root = buildBST(arr, n);
// 查找操作
BSTNode* node = search(root, 3);
if (node != NULL) {
cout << "查找到元素 " << node->data << endl;
} else {
cout << "未找到元素" << endl;
}
// 插入操作
root = insert(root, 10);
// 删除操作
root = remove(root, 5);
// 中序遍历打印
inorder(root);
return 0;
}
```
输出结果为:
```
查找到元素 3
1 2 3 4 6 7 8 9 10
```
其中,search()函数实现了查找操作,insert()函数实现了插入操作,buildBST()函数实现了建立操作,remove()函数实现了删除操作,inorder()函数实现了中序遍历打印操作。在主函数中,我们先建立了一个二叉排序树,然后对其进行了查找、插入、删除和中序遍历打印操作。
阅读全文
相关推荐











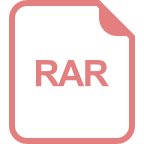





