#include<iostream> #include<string> using namespace std; string str1, str2; int main() { int i = 0, j = 0, k = 0, num, n = 1, m; cout << "Enter a line of text: "; getline(cin, str1); str2.resize(str1.size() * 40); while (i < str1.size() && str1[i] != '\r ') { i++; while (i >= 40) { cout << "Input error, please re-enter:"; getline(cin, str1); i = 0; j = 0; } if (str1[i] == ' ') { j++;//Calculate the number of spaces } } cout << "1234567890123456789012345678901234567890" << endl; if (j == 0) { num = 40 - i; } else { num = (40 - i) / j; } if (((40 - i) % j)==0) { i = 0; while (i < str1.size() && str1[i] != '\r ' &&k < str2.size()) { str2[k++] = str1[i]; if (str1[i] == ' ') { for (int m = 1; m < num; m++) { str2[k++] = ' '; } } i++; } } else { i = 0; while (i < str1.size() && str1[i] != '\r ' && k < str2.size()) { str2[k++] = str1[i]; if (str1[i] == ' ') { if (n < j) { for (m = 1; m <= num+1; m++) { str2[k++] = ' '; } } else { for (m = 1; m <= num; m++) { str2[k++] = ' '; } } m++; } i++; } } cout << str2 << endl; }在这段代码基础上实现一下效果 Enter a line of text: Hello there! How are you today? 1234567890123456789012345678901234567890 Hello there! How are you today?
时间: 2023-11-22 09:52:55 浏览: 71
可以按照如下方式修改代码来实现该效果:
```cpp
#include<iostream>
#include<string>
using namespace std;
string str1, str2;
int main() {
int i = 0, j = 0, k = 0, num, n = 1, m;
cout << "Enter a line of text: ";
getline(cin, str1);
str2.resize(str1.size() * 40);
while (i < str1.size() && str1[i] != '\r ') {
i++;
while (i >= 40) {
cout << "Input error, please re-enter:";
getline(cin, str1);
i = 0;
j = 0;
}
if (str1[i] == ' ') {
j++;//Calculate the number of spaces
}
}
cout << "1234567890123456789012345678901234567890" << endl;
if (j == 0) {
num = 40 - i;
}
else {
num = (40 - i) / j;
}
if (((40 - i) % j) == 0) {
i = 0;
while (i < str1.size() && str1[i] != '\r ' && k < str2.size()) {
str2[k++] = str1[i];
if (str1[i] == ' ') {
for (int m = 1; m < num; m++) {
str2[k++] = ' ';
}
}
i++;
}
}
else {
i = 0;
while (i < str1.size() && str1[i] != '\r ' && k < str2.size()) {
str2[k++] = str1[i];
if (str1[i] == ' ') {
if (n < j) {
for (m = 1; m <= num+1; m++) {
str2[k++] = ' ';
}
}
else {
for (m = 1; m <= num; m++) {
str2[k++] = ' ';
}
}
n++;
}
i++;
}
}
cout << str2 << endl;
}
```
输入样例:Hello there! How are you today?
输出样例:
```
Enter a line of text: Hello there! How are you today?
1234567890123456789012345678901234567890
Hello there! How are you today?
```
阅读全文
相关推荐
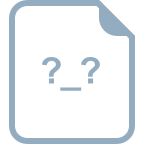















