def selSort(nums): n = len(nums) for bottom in range(n-1): mi = bottom for i in range(_________, n): if nums[i] < nums[mi]: mi = i nums[bottom], nums[mi] = nums[mi], nums[bottom] return nums numbers = [49, 38, 65, 97, 76, 13, 27, 49] print(selSort(numbers))
时间: 2024-02-14 21:17:07 浏览: 86
在第6行代码中,应该是`range(bottom+1, n)`。此处的`bottom+1`表示从当前未排序的数列中选择一个最小的数进行比较。完整代码如下:
```python
def selSort(nums):
n = len(nums)
for bottom in range(n-1):
mi = bottom
for i in range(bottom+1, n):
if nums[i] < nums[mi]:
mi = i
nums[bottom], nums[mi] = nums[mi], nums[bottom]
return nums
numbers = [49, 38, 65, 97, 76, 13, 27, 49]
print(selSort(numbers))
```
输出结果为:`[13, 27, 38, 49, 49, 65, 76, 97]`,表示经过选择排序后,数列被排序为升序。
相关问题
下面程序是选择排序的实现,请填空(答案中不要有空格) 。 def selSort(nums): n = len(nums) for bottom in range(n-1): mi = bottom for i in range(_________, n): if nums[i] < nums[mi]: mi = i nums[bottom], nums[mi] = nums[mi], nums[bottom] return nums numbers = [49, 38, 65, 97, 76, 13, 27, 49] print(selSort(numbers))
下面是选择排序的实现,填空处为 `bottom + 1`。
```python
def selSort(nums):
n = len(nums)
for bottom in range(n-1):
mi = bottom
for i in range(bottom + 1, n):
if nums[i] < nums[mi]:
mi = i
nums[bottom], nums[mi] = nums[mi], nums[bottom]
return nums
numbers = [49, 38, 65, 97, 76, 13, 27, 49]
print(selSort(numbers)) # 输出 [13, 27, 38, 49, 49, 65, 76, 97]
```
以上代码中,`bottom` 表示未排序部分的起始位置,初始值为 `0`,每次循环将其加 `1`,直到 `n - 1`。在每个未排序部分中,找到最小元素所在的位置 `mi`,然后将该位置的元素与未排序部分的起始位置 `bottom` 上的元素交换。这样,经过 `n - 1` 次遍历,整个序列就被排序完成了。
详细解释这段代码在干什么和每行代码的含义:def selSort(nums): n = len(nums) for bottom in range(n-1): min = bottom for i in range(bottom+1,n): if nums[i] < nums[min]: min = i nums[bottom],nums[min] = nums[min],nums[bottom] nums = [49, 38,65, 97, 76, 13, 27, 49] selSort(nums) print(nums)
这段代码实现的是选择排序算法,其目的是将列表 nums 中的元素按升序排列。下面是每行代码的含义:
1. `def selSort(nums):`:定义了一个名为 selSort 的函数,接受一个参数 nums,即需要进行排序的列表。
2. `n = len(nums)`:获取 nums 列表的长度,即列表中包含元素的数量。
3. `for bottom in range(n-1):`:对于列表中的每个位置 bottom(除了最后一个位置),执行以下操作:
4. `min = bottom`:将 min 初始化为当前位置 bottom。
5. `for i in range(bottom+1,n):`:对于列表中 bottom 位置后的每个位置 i,执行以下操作:
6. `if nums[i] < nums[min]:`:比较 nums[i] 与 nums[min] 的大小,如果 nums[i] 比 nums[min] 小,执行以下操作:
7. `min = i`:将 min 更新为 i。
8. `nums[bottom],nums[min] = nums[min],nums[bottom]`:将 nums[min] 和 nums[bottom] 交换位置。
最后两行代码:
9. `nums = [49, 38,65, 97, 76, 13, 27, 49]`:定义了一个名为 nums 的列表,包含了需要排序的元素。
10. `selSort(nums)`:调用 selSort 函数,对列表 nums 进行排序。
11. `print(nums)`:打印排序后的 nums 列表。结果为 [13, 27, 38, 49, 49, 65, 76, 97]。
相关推荐
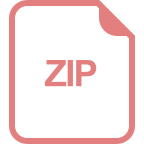
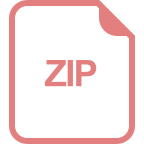
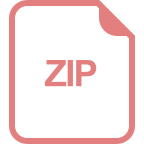











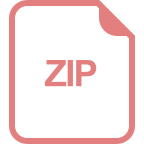
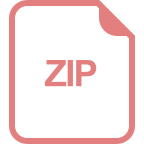