java解压zip文件以指定文件类型到本地
时间: 2023-05-29 11:01:21 浏览: 205
以下是使用Java解压zip文件并将指定文件类型保存到本地的示例代码:
```java
import java.io.*;
import java.util.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class UnzipExample {
public static void main(String[] args) throws IOException {
String zipFilePath = "path/to/zip/file.zip"; // zip文件路径
String fileType = ".txt"; // 要提取的文件类型
String outputFolderPath = "path/to/output/folder"; // 输出文件夹路径
unzipFiles(zipFilePath, fileType, outputFolderPath);
}
public static void unzipFiles(String zipFilePath, String fileType, String outputFolderPath) throws IOException {
File outputFolder = new File(outputFolderPath);
if (!outputFolder.exists()) {
outputFolder.mkdir();
}
InputStream inputStream = new FileInputStream(zipFilePath);
ZipInputStream zipInputStream = new ZipInputStream(inputStream);
ZipEntry zipEntry = zipInputStream.getNextEntry();
while (zipEntry != null) {
String fileName = zipEntry.getName();
if (fileName.toLowerCase().endsWith(fileType.toLowerCase())) {
String filePath = outputFolderPath + File.separator + fileName;
OutputStream outputStream = new FileOutputStream(filePath);
byte[] buffer = new byte[1024];
int length;
while ((length = zipInputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
outputStream.close();
}
zipEntry = zipInputStream.getNextEntry();
}
zipInputStream.closeEntry();
zipInputStream.close();
inputStream.close();
}
}
```
在该示例中,我们首先定义了要解压的zip文件的路径,要提取的文件类型和输出文件夹的路径。然后,将这些参数传递给`unzipFiles()`方法,该方法执行以下步骤:
1. 创建输出文件夹(如果还不存在)。
2. 读取zip文件并使用`ZipInputStream`逐个读取其条目。
3. 对于每个zip条目,检查其文件名是否以指定文件类型结尾。
4. 如果文件名匹配,则创建该文件的输出流,并将zip数据写入该文件。
5. 关闭输出流和zip条目。
6. 最后,关闭zip输入流和文件输入流。
请注意,该示例假定zip文件中的条目不包含文件夹,只包含文件。如果zip文件中包含文件夹,则需要相应地调整代码来处理它们。
阅读全文
相关推荐
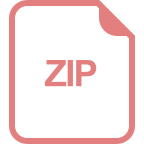
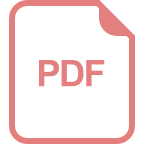
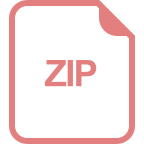
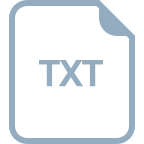
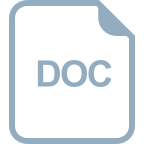
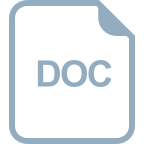
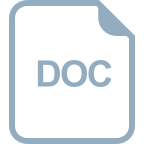
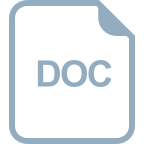
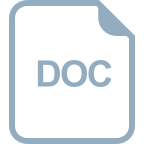
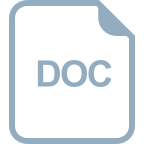
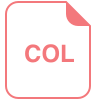

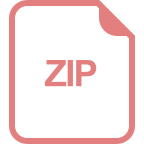
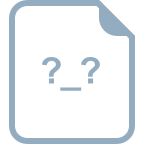
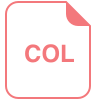
