某二叉排序树存在一个关键字x,在小于x的所有元素中,查找值最大的元素并返回该节点。解题思路:中序遍历该树找x结点前驱,借助前驱指针pre。给出完整代码并详细解释。用C语言给出并且用迭代的方式
时间: 2024-10-25 11:10:44 浏览: 17
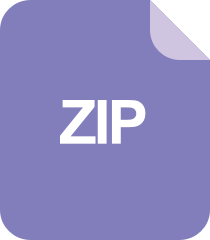
二叉排序树_17281183_刘梦婷_leavingtbk_二叉排序树_

在二叉排序树中找到小于给定关键字 `x` 的最大元素,可以采用迭代的方式来实现,主要是通过中序遍历来找到这个节点。因为中序遍历会先访问左子树(所有小于当前节点的元素),然后访问根节点,最后访问右子树。
首先,我们需要定义一个辅助结构体 `Node` 来表示树中的节点,并维护一个指向前驱节点的指针 `pre`:
```c
typedef struct Node {
int key;
struct Node *left, *right;
struct Node *pre; // 前驱节点指针
} Node;
// 创建新节点
Node* newNode(int key) {
Node* node = (Node*)malloc(sizeof(Node));
node->key = key;
node->left = node->right = NULL;
node->pre = NULL; // 初始前驱为NULL
return node;
}
// 插入节点,这里假设树已存在
void insert(Node** root, int key) {
if (*root == NULL) {
*root = newNode(key);
} else {
Node* current = *root;
while (current != NULL) {
pre = current->pre;
if (key < current->key) {
current->pre = newNode(key); // 将新节点插入到合适位置
if (pre != NULL) {
pre->right = current->pre;
}
else {
*root = current->pre;
}
break;
} else {
pre = current;
current = current->left;
}
}
}
}
```
接下来,我们编写一个函数 `findMaxLessThanX` 来寻找小于 `x` 的最大元素:
```c
Node* findMaxLessThanX(Node* root, int x) {
Node* max_less_x = NULL; // 初始化为NULL
Node* current = root;
// 使用迭代实现中序遍历
while (current != NULL) {
if (current->key < x) {
if (max_less_x == NULL || max_less_x->key < current->key) {
max_less_x = current; // 更新最大元素为当前节点
}
current = current->right; // 继续向右搜索
}
else {
current = current->pre; // 当前节点大于x,回溯到其前驱
}
}
return max_less_x;
}
```
现在我们可以使用 `insert` 函数先构建二叉排序树,然后通过 `findMaxLessThanX` 函数找到小于 `x` 的最大元素。注意在实际应用中,你需要处理空树的情况以及插入操作。
阅读全文
相关推荐
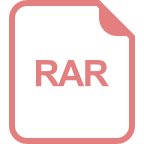
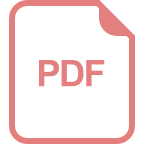
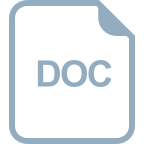
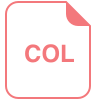


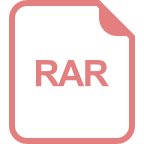
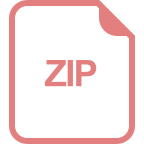
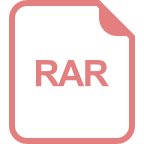
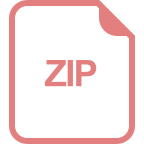
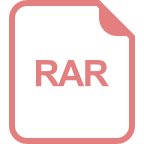
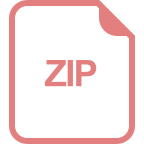
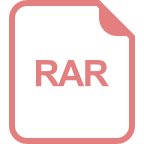
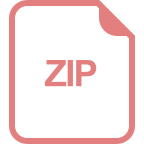
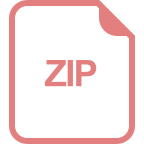