FileChannel 加密
时间: 2024-05-17 08:18:54 浏览: 86
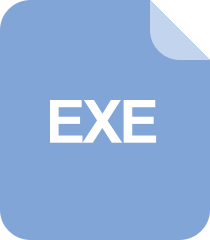
文件加密
要对 FileChannel 进行加密,你可以使用 Java 的加密工具来实现。以下是一个简单的示例代码,演示了如何使用 AES 加密算法对文件进行加密和解密。
```java
import javax.crypto.*;
import javax.crypto.spec.SecretKeySpec;
import java.io.*;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class FileEncryption {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
// 生成密钥
private static SecretKey generateKey(String encryptionKey) throws NoSuchAlgorithmException {
return new SecretKeySpec(encryptionKey.getBytes(), ALGORITHM);
}
// 加密文件
public static void encryptFile(String sourceFilePath, String destinationFilePath, String encryptionKey)
throws IOException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException {
SecretKey secretKey = generateKey(encryptionKey);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
try (FileInputStream inputStream = new FileInputStream(sourceFilePath);
FileOutputStream outputStream = new FileOutputStream(destinationFilePath);
FileChannel inputChannel = inputStream.getChannel();
FileChannel outputChannel = outputStream.getChannel()) {
ByteBuffer buffer = ByteBuffer.allocateDirect(1024);
while (inputChannel.read(buffer) != -1) {
buffer.flip();
byte[] bytes = new byte[buffer.limit()];
buffer.get(bytes);
byte[] encryptedBytes = cipher.update(bytes);
outputChannel.write(ByteBuffer.wrap(encryptedBytes));
buffer.clear();
}
byte[] finalEncryptedBytes = cipher.doFinal();
outputChannel.write(ByteBuffer.wrap(finalEncryptedBytes));
} catch (IOException | ShortBufferException | IllegalBlockSizeException | BadPaddingException e) {
e.printStackTrace();
throw new IOException("Error encrypting file", e);
}
}
// 解密文件
public static void decryptFile(String sourceFilePath, String destinationFilePath, String encryptionKey)
throws IOException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException {
SecretKey secretKey = generateKey(encryptionKey);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
try (FileInputStream inputStream = new FileInputStream(sourceFilePath);
FileOutputStream outputStream = new FileOutputStream(destinationFilePath);
FileChannel inputChannel = inputStream.getChannel();
FileChannel outputChannel = outputStream.getChannel()) {
ByteBuffer buffer = ByteBuffer.allocateDirect(1024);
while (inputChannel.read(buffer) != -1) {
buffer.flip();
byte[] bytes = new byte[buffer.limit()];
buffer.get(bytes);
byte[] decryptedBytes = cipher.update(bytes);
outputChannel.write(ByteBuffer.wrap(decryptedBytes));
buffer.clear();
}
byte[] finalDecryptedBytes = cipher.doFinal();
outputChannel.write(ByteBuffer.wrap(finalDecryptedBytes));
} catch (IOException | ShortBufferException | IllegalBlockSizeException | BadPaddingException e) {
e.printStackTrace();
throw new IOException("Error decrypting file", e);
}
}
public static void main(String[] args) {
String sourceFilePath = "path/to/source/file";
String encryptedFilePath = "path/to/encrypted/file";
String decryptedFilePath = "path/to/decrypted/file";
String encryptionKey = "yourEncryptionKey";
try {
encryptFile(sourceFilePath, encryptedFilePath, encryptionKey);
decryptFile(encryptedFilePath, decryptedFilePath, encryptionKey);
} catch (IOException | NoSuchAlgorithmException | NoSuchPaddingException | InvalidKeyException e) {
e.printStackTrace();
}
}
}
```
在上述示例代码中,你需要用实际的文件路径替换 `sourceFilePath`、`encryptedFilePath` 和 `decryptedFilePath` 的占位符,并设置一个用于加密和解密的密钥 `encryptionKey`。该代码使用 AES 算法和 ECB 模式进行加密和解密。请注意,ECB 模式在某些情况下可能存在安全隐患,因此你可以选择其他更安全的模式,例如 CBC 模式。
该示例使用了 Java 的 NIO 文件通道(FileChannel)来读取和写入文件数据。加密和解密过程通过对文件数据进行分块处理,每次处理一个缓冲区(ByteBuffer),并将加密或解密后的数据写入输出文件。
请确保在使用此代码时仔细阅读并理解其中的逻辑,并根据自己的需求进行适当的修改和优化。
阅读全文
相关推荐
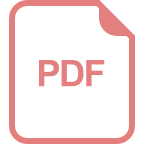
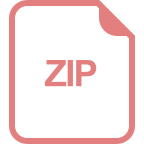
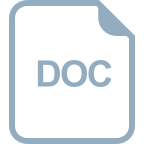
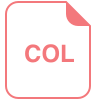
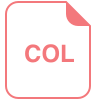
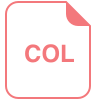


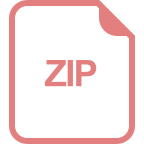
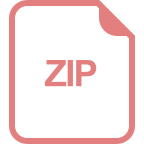
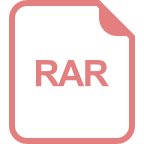
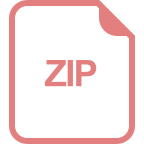
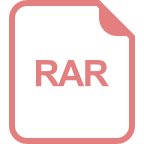
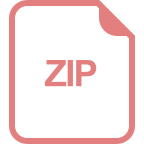