face_enhancer
时间: 2024-07-29 08:01:36 浏览: 114
Face Enhancer通常指的是图像处理软件或工具,用于增强或美化人脸图片。它可能包括的功能有:
1. **面部修饰**:可以校正对齐、去除瑕疵(如红眼、痘痘)、提亮肤色等[^4]。
```python
from PIL import Image, ImageEnhance
# 假设im是处理后的Image对象
enhancer = ImageEnhance.Brightness(im)
brightened_im = enhancer.enhance(1.2) # 提亮10%
```
2. **美颜效果**:应用滤镜、磨皮、柔焦等效果[^5]。
```python
from skimage import img_as_float
from skimage.filter import gaussian_filter
# 对图像应用模糊效果
filtered_im = img_as_float(im)
filtered_im = gaussian_filter(filtered_im, sigma=2)
```
3. **智能美容**:利用AI技术识别并修复皱纹、痘痕等细节[^6]。
请注意,实际操作取决于所使用的具体工具或库。如果你正在寻找一个特定的工具,可能需要查看其官方文档或教程。
相关问题
Java Enhancer
Java Enhancer 是一个 Java 字节码增强工具,它可以在编译期或运行期对 Java 类进行增强,以实现一些特定的功能,比如 AOP、事务管理等。它可以通过修改字节码来实现这些功能,而不需要修改源代码。
java Enhancer
Java Enhancer 是一个用于动态生成 Java 类的工具,它可以通过字节码增强技术,为现有的 Java 类添加新的功能或修改现有的功能。它通常用于 AOP(面向切面编程)框架中,例如 Spring AOP。
相关推荐
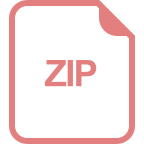
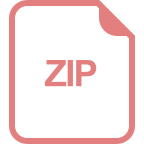
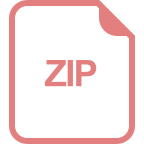













