已知两个有公共结点的无头结点的单向链表(如下图所示),编写程序,找出这两个单链表的第一个公共结
时间: 2024-09-30 10:14:25 浏览: 41
已知两个有公共节点的无头结点单向链表,寻找它们的第一个公共结点的问题通常通过“快慢指针”算法来解决。在这个算法中,我们维护两个指针,一个移动得较快(通常是原地步进两步),另一个移动较慢(一步)。当快指针追上慢指针时,说明它们已经相遇过一次,此时慢指针所在的位置就是公共部分的起点。如果快指针还没有超过链表长度,则继续让慢指针前进,直到再次追上快指针,这就是第一个公共节点。
以下是Python的一个简单实现:
```python
def findFirstCommonNode(list1, list2):
if not list1 or not list2: return None # 如果其中一个链表为空,直接返回None
slow_ptr1 = list1.head
slow_ptr2 = list2.head
fast_ptr1 = list1.head
fast_ptr2 = list2.head
while fast_ptr1 and fast_ptr1 != slow_ptr2:
fast_ptr1 = fast_ptr1.next if fast_ptr1 else None
fast_ptr2 = fast_ptr2.next if fast_ptr2 else None
slow_ptr2 = slow_ptr2.next if slow_ptr2 else None
if fast_ptr1 is None or fast_ptr2 is None or fast_ptr1 != fast_ptr2:
return None # 指针未交叉则无公共节点
# 现在找到交点,从交点开始遍历,直到找到相同的节点
common_ptr = fast_ptr1
while common_ptr and common_ptr.next:
common_ptr1 = list1.head
common_ptr2 = list2.head
while common_ptr1 and common_ptr1 != common_ptr:
common_ptr1 = common_ptr1.next
while common_ptr2 and common_ptr2 != common_ptr:
common_ptr2 = common_ptr2.next
if common_ptr1 == common_ptr2:
return common_ptr
common_ptr = common_ptr.next
return None # 没有找到公共节点
# 使用示例:
# 假设list1和list2的头结点分别为node1和node2,这里省略实际链表创建过程
first_common_node = findFirstCommonNode(list1, list2)
```
阅读全文
相关推荐
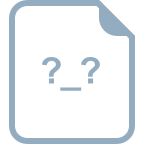
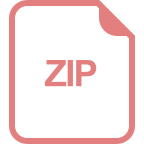
















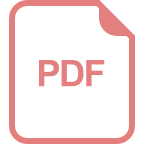