在单链表指定位置插入一个节点
时间: 2024-10-29 22:09:10 浏览: 8
在单链表中,在指定位置插入一个节点通常涉及到以下几个步骤:
1. **创建新节点**:首先,你需要创建一个新的节点,该节点的数据部分存储你要插入的值。
2. **定位目标位置**:确定你想在当前链表中的哪个位置插入。这需要遍历链表,从头节点开始计数,直到找到第`n`个节点(其中`n`是插入位置)。
3. **连接节点**:找到了目标位置之后,将新节点的`next`指针指向当前目标节点的下一个节点。如果目标位置是第一个节点(即头节点),那么新节点的`next`应该指向原来的头节点。
4. **更新引用**:如果目标位置不是最后一个节点,那么目标节点的`next`指针应当指向新插入的节点;如果是最后一个节点,则无需改变其`next`指针。
5. **结束操作**:完成上述步骤后,新的节点就在指定位置插入到链表中了。
下面是一个简单的Python示例,假设我们有一个Node类和一个LinkedList类:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def insert_at(self, position, value):
new_node = Node(value)
if position == 0: # 插入在头部
new_node.next = self.head
self.head = new_node
else:
current = self.head
for _ in range(position - 1): # 遍历到目标位置前一个节点
if current is not None:
current = current.next
if current is not None: # 如果找到位置,插入新节点
new_node.next = current.next
current.next = new_node
```
阅读全文
相关推荐
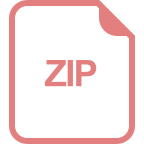
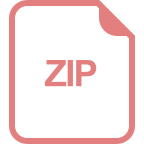
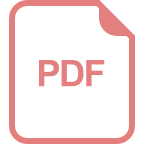




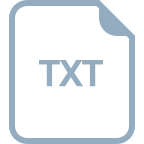
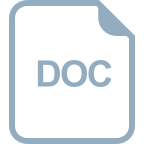
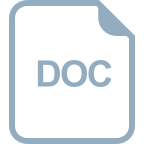
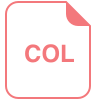







