不用任何库,用c语言实现正切函数计算
时间: 2024-09-23 22:07:18 浏览: 49
在C语言中,为了实现正切函数(tan),你需要利用数学库`math.h`中的函数`tanh()`。因为`tanh()`实际上是一个双曲正切函数,它对`atanh()`的输入进行转换后再计算出正切值。如果你需要精确的正切计算而不想依赖库函数,可以使用泰勒级数展开法来近似计算,但这个过程比较复杂并且精度受限于级数项的选取。
以下是简单使用`math.h`库的示例:
```c
#include <stdio.h>
#include <math.h>
double calculate_tan(double angle) {
return tan(angle);
}
int main() {
double input_angle;
printf("请输入角度:");
scanf("%lf", &input_angle);
double result = calculate_tan(input_angle);
printf("正切值为: %lf\n", result);
return 0;
}
```
如果你想自定义实现,一个简单的泰勒级数方法会涉及逐次加减多项式,但这超出了本平台提供的支持范围,建议查阅相关的数学文献或教程来进行详细学习。
相关问题
不用math.h库,用c语言实现正切函数
如果不用 `math.h` 库直接在 C 语言中实现正切函数,可以使用泰勒级数(Taylor Series)的方式近似计算。然而,这种方法对于复杂的数学运算来说效率不高,适合教学演示,而不是实际生产环境。
下面是一个简单的泰勒级数实现正切的例子,只适用于小角度(小于 π/4):
```c
#include <stdio.h>
// 定义一个辅助函数,用于递归计算泰勒级数
double taylor_term(int n, double x) {
if (n % 2 == 0)
return pow(-x, n) / factorial(n);
else
return pow(x, n - 1) / factorial(n - 1);
}
// 计算阶乘
unsigned long long factorial(int n) {
unsigned long long fact = 1;
for (int i = 2; i <= n; ++i)
fact *= i;
return fact;
}
double my_tan(double angle) {
const int terms = 10; // 使用的级数项数量,越多越精确
double sum = 0;
for (int i = 1; i <= terms; ++i) {
double term = taylor_term(i, angle);
sum += term;
}
return sum;
}
int main() {
double angle;
printf("请输入一个小于π/4的角度:");
scanf("%lf", &angle);
double result = my_tan(angle);
printf("角度 %.2f 的正切值(近似)为:%.8lf\n", angle, result);
return 0;
}
```
这个程序实现了基于有限项的泰勒级数,但请注意这并不是最准确或最高效的算法,仅限于了解原理。实际应用中还是建议使用 `math.h` 中的 `tan()` 函数,因为它们已经优化过了。
如何用c语言实现三角函数
C语言中提供了一些数学库函数,包括三角函数,可以使用这些函数来计算三角函数的值。下面是一些常用的三角函数及其对应的C语言函数:
- sin(x):计算正弦函数的值,可使用 math.h 头文件中的 sin 函数。
- cos(x):计算余弦函数的值,可使用 math.h 头文件中的 cos 函数。
- tan(x):计算正切函数的值,可使用 math.h 头文件中的 tan 函数。
- asin(x):计算反正弦函数的值,可使用 math.h 头文件中的 asin 函数。
- acos(x):计算反余弦函数的值,可使用 math.h 头文件中的 acos 函数。
- atan(x):计算反正切函数的值,可使用 math.h 头文件中的 atan 函数。
这些函数的参数都是以弧度为单位的角度值。如果要使用以度为单位的角度值,需要先将角度值转换为弧度值,例如:
```c
#include <math.h>
#define PI 3.14159265
double degree_to_radian(double degree) {
return degree * PI / 180.0;
}
```
然后可以用以下方式来计算三角函数的值:
```c
#include <math.h>
double x = 1.0;
double sin_value = sin(x);
double cos_value = cos(x);
double tan_value = tan(x);
double asin_value = asin(x);
double acos_value = acos(x);
double atan_value = atan(x);
```
需要注意的是,由于计算机使用的是有限精度的浮点数表示,因此在计算三角函数时可能会产生一些误差。
阅读全文
相关推荐
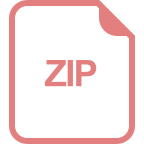
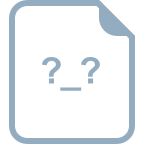
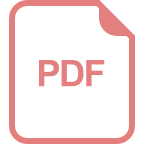
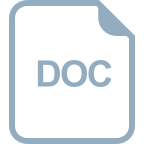
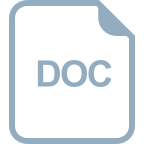
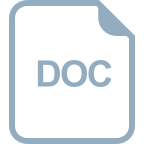
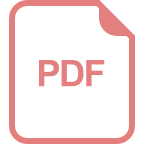
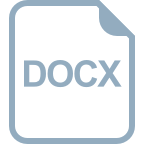
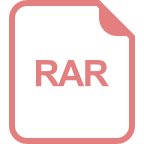
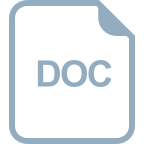
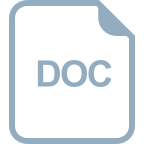
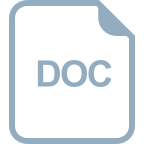
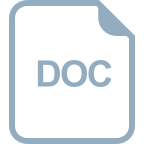
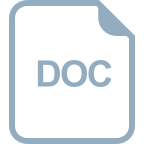
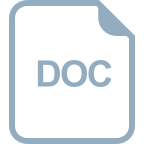
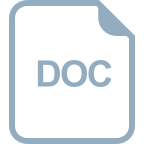
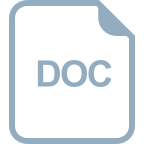