食谱库:提供丰富的健康食谱资源,按照膳食需求分类(如低脂、高蛋白、素食等),并可以搜索、添加和收藏。Java代码
时间: 2024-09-25 13:10:40 浏览: 32
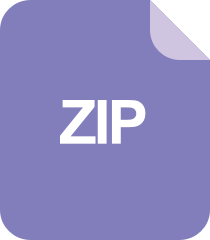
MyMealPrep:一种应用程序,可用于搜索食谱以及创建膳食计划并基于您的膳食计划所选食谱生成购物清单的功能
在Java中,构建一个食谱库系统通常会涉及到数据库操作、RESTful API的设计以及前端展示。这里简述一下基本的数据库模型和部分API接口:
1. 数据库模型(使用JPA):
```java
@Entity
public class Recipe {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String description;
private String dietType; // 低脂、高蛋白、素食等
private String imageUrl;
private List<String> ingredients;
// getter和setter方法
}
```
2. Repository接口:
```java
@Repository
public interface RecipeRepository extends JpaRepository<Recipe, Long> {
List<Recipe> findRecipesByDietType(String dietType);
}
```
3. 服务类(例如,RestController):
```java
@RestController
@RequestMapping("/api/recipes")
public class RecipeController {
@Autowired
private RecipeRepository recipeRepository;
@GetMapping("/{dietType}")
public ResponseEntity<List<Recipe>> getRecipesByDietType(@PathVariable String dietType) {
List<Recipe> recipes = recipeRepository.findRecipesByDietType(dietType);
return new ResponseEntity<>(recipes, HttpStatus.OK);
}
@PostMapping
public Recipe createRecipe(@Valid @RequestBody Recipe recipe) {
recipeRepository.save(recipe);
return recipe;
}
// 添加搜索、收藏API方法
}
```
4. 前端部分(HTML+JavaScript):
- 搜索功能:通过AJAX向服务器发送GET请求,传入搜索关键字。
- 添加和收藏:用户提交表单时发送POST请求到相应的API。
注意,这是一个基础示例,实际项目还需要处理异常、错误处理、分页显示、认证授权等。同时,为了安全性,应尽量避免直接在前端展示敏感信息,如密码。
阅读全文
相关推荐
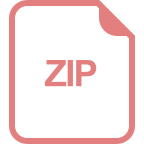
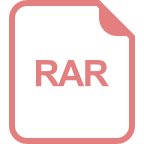
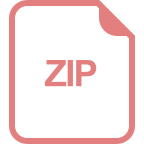
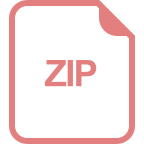
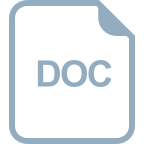
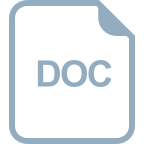
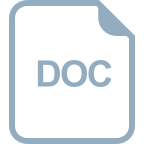
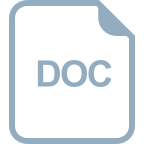
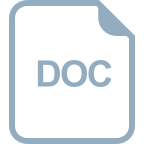
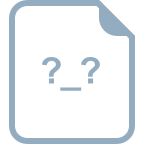
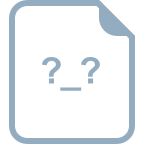