用C++,只用输入一个字符串,一共有两种功能,解密和加密的程序
时间: 2024-11-25 12:17:31 浏览: 5
在C++中,创建一个简单的字符串解密和加密程序,可以使用常见的替换密码技术,比如Caesar Cipher(凯撒密码),通过固定偏移量将字母表中的字符移动。这里我们可以设计两个函数:`encrypt`和`decrypt`。
```cpp
#include <iostream>
#include <string>
// 函数声明
std::string encrypt(const std::string& input, int shift);
std::string decrypt(const std::string& input, int shift);
int main() {
std::string message;
std::cout << "请输入字符串 (加密/解密模式选择): ";
getline(std::cin, message);
// 检查用户是否选择了加密或解密
if (message == "加密") {
int shift;
std::cout << "请输入偏移量: ";
std::cin >> shift;
message = encrypt(message, shift);
std::cout << "加密后的字符串: " << message << "\n";
} else if (message == "解密") {
int shift;
std::cout << "请输入偏移量: ";
std::cin >> shift;
message = decrypt(message, -shift); // 使用负数进行解密操作
std::cout << "解密后的字符串: " << message << "\n";
} else {
std::cout << "无效的选择,请输入'加密'或'解密'。\n";
return 0;
}
return 0;
}
// Caesar Cipher 实现
std::string encrypt(const std::string& input, int shift) {
std::string result = "";
for (char c : input) {
if ('a' <= c && c <= 'z') { // 只处理小写字母
result += static_cast<char>((c + shift - 'a') % 26 + 'a');
} else if ('A' <= c && c <= 'Z') {
result += static_cast<char>((c + shift - 'A') % 26 + 'A');
} else {
result += c; // 非字母字符不变
}
}
return result;
}
std::string decrypt(const std::string& input, int shift) {
return encrypt(input, -shift);
}
```
在这个程序中,用户可以选择加密还是解密,并提供一个偏移量作为密钥。注意,这个示例仅限于英文字符(大小写字母),对于其他语言或特殊字符可能会需要更复杂的处理。
阅读全文
相关推荐
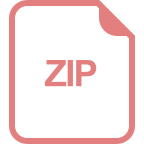
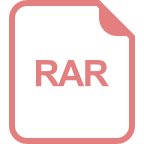
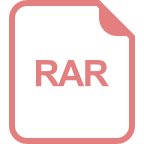
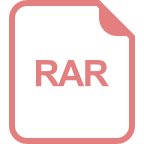






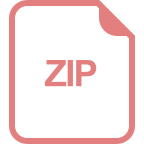
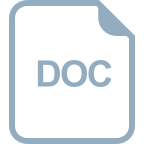
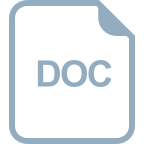
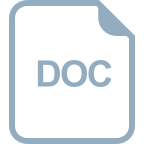
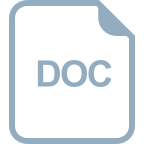
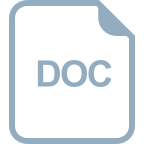
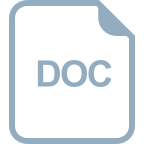
