c++ auto遍历
时间: 2024-01-04 08:36:40 浏览: 33
C++11引入了auto关键字,它可以用于自动推断变量的类型。在遍历容器时,使用auto关键字可以简化代码。下面是一个示例:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
```
在这个示例中,我们使用auto关键字来推断迭代器的类型,并使用它来遍历numbers容器。在每次迭代中,我们使用解引用运算符*来访问当前迭代器指向的元素。
请注意,auto关键字在C++11之前是不可用的,如果你使用的是旧版本的C++编译器,则需要手动指定迭代器的类型。
相关问题
c++ auto遍历时提示表达式必须包含指针类型
这个错误通常是因为您在使用 auto 关键字遍历时,表达式的类型不是指针类型。auto 关键字会根据等号右边的表达式来自动推断变量的类型,如果表达式的类型不是指针类型,就会出现这个错误。
例如,如果您想要使用 auto 遍历一个数组,应该这样写:
```
int arr[] = {1, 2, 3, 4, 5};
for(auto &x : arr) {
// do something with x
}
```
如果您想要使用 auto 遍历一个指针数组,应该这样写:
```
int* arr[] = {new int(1), new int(2), new int(3)};
for(auto &x : arr) {
// do something with x
}
```
如果您想要使用 auto 遍历一个 vector 的元素,应该这样写:
```
std::vector<int> vec = {1, 2, 3, 4, 5};
for(auto &x : vec) {
// do something with x
}
```
请注意,如果您遍历的对象是一个指针类型,您需要使用引用(&)来确保您可以修改遍历到的对象。
c++map遍历auto
C++中的std::map是一个关联容器,它提供了一种将键值对映射起来的方式。如果你想要遍历一个std::map并使用auto关键字来推断迭代器的类型,你可以使用范围遍历循环(range-based for loop)进行遍历操作。下面是一个示例代码:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
在这个例子中,我们创建了一个std::map对象myMap,并给它添加了三对键值对。然后,我们使用范围遍历循环来遍历整个map。循环中的每个元素都被绑定到名为pair的变量中,其中pair.first表示键,pair.second表示值。我们使用auto关键字来推断迭代器的类型,并使用const修饰符来确保不修改元素。
输出结果为:
```
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
这样就完成了对std::map的遍历操作。希望能帮到你!如果还有其他问题,请继续提问。
相关推荐
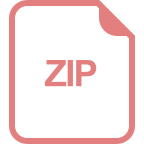
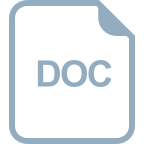
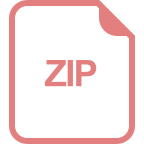
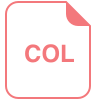
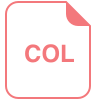










