point.add chart
时间: 2024-09-07 11:04:51 浏览: 23
"point.add chart"这个表述似乎是在谈论将数据点添加到图表的操作。在数据可视化或编程中,"point"通常指数据系列中的一个标记或值,而"chart"则代表用于展示数据的图形,比如折线图、柱状图或散点图等。
如果你想要在一个程序中创建这样的操作,例如在Python的Matplotlib库中,你可以这样做:
```python
import matplotlib.pyplot as plt
# 创建一个数据点列表
data_points = [1, 2, 3, 4, 5]
# 创建一个新的图表
plt.figure()
line, = plt.plot(data_points)
# 添加数据点到图表上
for point in data_points:
line.set_data(range(1, len(point) + 1), point)
plt.draw() # 更新图表显示
```
在这个例子中,`add`函数并不是直接针对`point`和`chart`的,而是通过动态更新数据并调用`set_data`方法将每个数据点添加到已经创建好的线(`line`)上。
相关问题
// 清空所有的Series和Points chart1.Series.Clear(); chart1.Palette = ChartColorPalette.Pastel; chart1.Titles.Add("用户人数统计"); // 添加一个名为"Users"的Series Series series = chart1.Series.Add("Users"); series.ChartType = SeriesChartType.Pie; // 添加数据点 string constr = SqlHelper.sqlcon; NpgsqlConnection conn = new NpgsqlConnection(constr); NpgsqlDataAdapter AdapterSelect = new NpgsqlDataAdapter(); conn.Open(); NpgsqlCommand sqlCmd1 = new NpgsqlCommand(); string sql1 = "select count(*) from login where isAdmin = '1' "; //显示管理员人数 sqlCmd1 = new NpgsqlCommand(sql1, conn); object result1 = sqlCmd1.ExecuteScalar(); NpgsqlCommand sqlCmd2 = new NpgsqlCommand(); string sql2 = "select count(*) from login where isAdmin = '0' "; //显示一般用户人数 sqlCmd1 = new NpgsqlCommand(sql2, conn); object result2 = sqlCmd1.ExecuteScalar(); series.Points.AddXY("一般用户", result2); series.Points.AddXY("管理员", result1); // 设置标签和颜色 series.LabelFormat = "{0}%"; foreach (DataPoint point in series.Points) { point.Label = "#VALX: #VALY"; if (point.AxisLabel == "一般用户") point.Color = Color.Green; else if (point.AxisLabel == "管理员") point.Color = Color.Red; }百分数未显示
在这段代码中,设置了`series.LabelFormat = "{0}%"`来设置标签格式为百分数,但是在循环中设置了点的标签为`point.Label = "#VALX: #VALY"`,这会覆盖掉之前设置的百分数格式,导致标签中不能正确显示百分数。
解决方法是将循环中设置标签的代码注释掉或删除,这样就能正确显示百分数了。代码修改如下:
```
// 清空所有的Series和Points
chart1.Series.Clear();
chart1.Palette = ChartColorPalette.Pastel;
chart1.Titles.Add("用户人数统计");
// 添加一个名为"Users"的Series
Series series = chart1.Series.Add("Users");
series.ChartType = SeriesChartType.Pie;
// 添加数据点
string constr = SqlHelper.sqlcon;
NpgsqlConnection conn = new NpgsqlConnection(constr);
NpgsqlDataAdapter AdapterSelect = new NpgsqlDataAdapter();
conn.Open();
NpgsqlCommand sqlCmd1 = new NpgsqlCommand();
string sql1 = "select count(*) from login where isAdmin = '1' "; //显示管理员人数
sqlCmd1 = new NpgsqlCommand(sql1, conn);
object result1 = sqlCmd1.ExecuteScalar();
NpgsqlCommand sqlCmd2 = new NpgsqlCommand();
string sql2 = "select count(*) from login where isAdmin = '0' "; //显示一般用户人数
sqlCmd2 = new NpgsqlCommand(sql2, conn);
object result2 = sqlCmd2.ExecuteScalar();
series.Points.AddXY("一般用户", result2);
series.Points.AddXY("管理员", result1);
// 设置标签和颜色
series.LabelFormat = "{0}%";
foreach (DataPoint point in series.Points)
{
// 将下面这行代码注释掉或删除
// point.Label = "#VALX: #VALY";
if (point.AxisLabel == "一般用户")
point.Color = Color.Green;
else if (point.AxisLabel == "管理员")
point.Color = Color.Red;
}
```
r语言这个图大小改大一点 #test #wujun的包 # 构造示例数据 data <- tibble( name = c('Citizen', 'Work Permit', 'Permanent Resident'), y = c(73,17,3)) highchart() %>% hc_chart(type = "pie",backgroundColor='#ffffff') %>% hc_add_series(data = data, colorByPoint = TRUE, innerSize = '50%') %>% hc_tooltip(pointFormat = '{series.name}: <b>{point.percentage:.1f}%</b>') %>% hc_add_theme(hc_theme_flat()) %>% hc_legend( #图例标题 title = list(text = "图例"), #图例是否显示 enabled = TRUE, #图例布局位置 align = "right", verticalAlign = "top", #是否浮动:图例是否在图内 floating = TRUE, #垂直或水平摆放 layout = "vertical", #图例的相对位置 x = 0, y =0)%>% hc_plotOptions(pie = list( allowPointSelect = TRUE, cursor = 'pointer', dataLabels = list( enabled = T, format = '<b>{point.name}</b>: {point.percentage:.1f} %' ), showInLegend = T, startAngle = -90, # 圆环的开始角度 endAngle = 90, # 圆环的结束角度 center = c('50%', '50%') ))
你可以通过增加`hc_chart`中的`height`和`width`参数来调整图形大小。例如,将`height`和`width`都设置为500,代码如下:
```
library(highcharter)
library(tibble)
# 构造示例数据
data <- tibble(
name = c('Citizen', 'Work Permit', 'Permanent Resident'),
y = c(73,17,3)
)
highchart() %>%
hc_chart(type = "pie", backgroundColor='#ffffff', height = 500, width = 500) %>%
hc_add_series(data = data,
colorByPoint = TRUE,
innerSize = '50%') %>%
hc_tooltip(pointFormat = '{series.name}: <b>{point.percentage:.1f}%</b>') %>%
hc_add_theme(hc_theme_flat()) %>%
hc_legend(
#图例标题
title = list(text = "图例"),
#图例是否显示
enabled = TRUE,
#图例布局位置
align = "right", verticalAlign = "top",
#是否浮动:图例是否在图内
floating = TRUE,
#垂直或水平摆放
layout = "vertical",
#图例的相对位置
x = 0, y =0) %>%
hc_plotOptions(pie = list(
allowPointSelect = TRUE,
cursor = 'pointer',
dataLabels = list(
enabled = T,
format = '<b>{point.name}</b>: {point.percentage:.1f} %'
),
showInLegend = T,
startAngle = -90, # 圆环的开始角度
endAngle = 90, # 圆环的结束角度
center = c('50%', '50%')
))
```
你可以根据需要调整`height`和`width`的值。
阅读全文
相关推荐
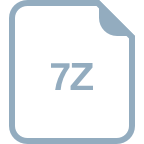
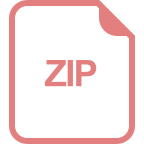
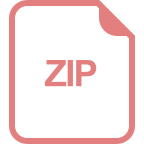

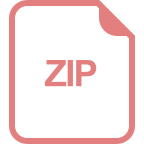
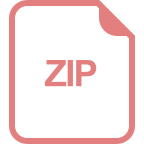
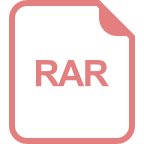
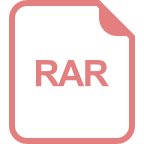
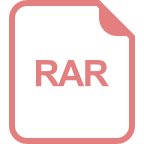
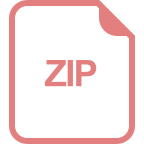
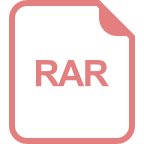
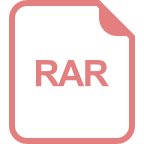
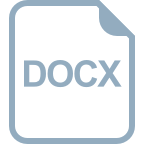



