QPixmap bufferPixmap(size()); bufferPixmap.fill(Qt::transparent); if(_openflag == 0)//不是打开图片的,每一次新建一个空白的画布 { _pixmap = QPixmap(size());//新建pixmap _pixmap.fill(Qt::white);//背景色填充为白色 } QPixmap pix = _pixmap;//以_pixmap作为画布 QPainter p(&pix);//将_pixmap作为画布 QPainter p1(&bufferPixmap); unsigned int i1=0,i2=0,i3=0,i4=0;//各种图形的索引 unsigned int i5=0,i6=0,i7=0,i8=0;//各种图形的索引 Q_UNUSED(event); QPen pen; pen.setColor(color); p.setPen(pen); for(int c = 0;c<_shape.size();++c)//控制用户当前所绘图形总数 { if(_shape.at(c) == 1)//线条 { const QVector<QPoint>& line = _lines.at(i1++);//取出一条线条 for(int j=0; j<line.size()-1; ++j)//将线条的所有线段描绘出 { p.drawLine(line.at(j), line.at(j+1)); } } else if(_shape.at(c) == 2)//矩形 { p.drawRect(_rects.at(i2++)); } else if(_shape.at(c) == 3)//椭圆 { p.drawEllipse(_ellipse.at(i3++)); } else if(_shape.at(c) == 4)//直线 { p.drawLine(_line.at(i4).topLeft(),_line.at(i4).bottomRight()); i4++; } } if(drawFinished) { for(int c = 0;c<_shape.size();++c)//控制用户当前所绘图形总数 { if(_shape.at(c) == 1)//线条 { const QVector<QPoint>& line = _lines.at(i5++);//取出一条线条 for(int j=0; j<line.size()-1; ++j)//将线条的所有线段描绘出 { p1.drawLine(line.at(j), line.at(j+1)); } } else if(_shape.at(c) == 2)//矩形 { p1.drawRect(_rects.at(i6++)); } else if(_shape.at(c) == 3)//椭圆 { p1.drawEllipse(_ellipse.at(i7++)); } else if(_shape.at(c) == 4)//直线 { p1.drawLine(_line.at(i4).topLeft(),_line.at(i4).bottomRight()); i8++; } } p1.end(); } p.end();//结束对pix的绘制 p.begin(this); // 将当前窗体作为画布,开始对当前窗体进行绘制 p.drawPixmap(0, 0, bufferPixmap); p.drawPixmap(0, 0, pix);
时间: 2024-04-12 09:32:54 浏览: 176
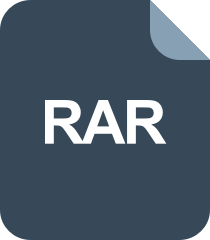
qt_browser.rar_GDI/图象编程_QT_
这段代码是一个绘图相关的代码片段,主要是使用Qt框架进行图形的绘制。首先,通过创建一个空白的画布`bufferPixmap`,并用透明色进行填充。然后根据`_openflag`的值来判断是否需要新建一个空白的画布`_pixmap`,并将其背景色填充为白色。
接下来,使用`QPainter`类将`_pixmap`作为画布进行绘制操作。根据`_shape`中的值来确定绘制的图形类型,通过不同的条件分支来绘制线条、矩形、椭圆和直线等图形。其中,线条通过遍历`_lines`中的点来绘制,矩形通过`_rects`中的矩形参数进行绘制,椭圆通过`_ellipse`中的椭圆参数进行绘制,直线通过`_line`中的起始点和结束点进行绘制。
最后,根据`drawFinished`的值来决定是否在`bufferPixmap`上进行绘制。同样地,根据`_shape`的值来确定绘制的图形类型,并通过条件分支来绘制线条、矩形、椭圆和直线等图形。
最后,通过`QPainter`类将绘制好的图像分别绘制在当前窗体上,使用`drawPixmap()`方法设置位置和大小。
阅读全文
相关推荐
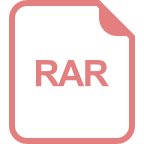
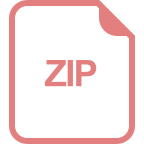















