输出123456789怎么变成123
时间: 2024-11-25 14:32:37 浏览: 3
如果想要将连续的数字"123456789"截断到只显示前三位,可以使用字符串切片或者循环截取的方式来实现。这里是几种常见编程语言的例子:
**Python:**
```python
number_string = "123456789"
shortened_number = number_string[:3]
print(shortened_number) # 输出: 123
```
**Java:**
```java
String numberString = "123456789";
int length = 3;
String shortenedNumber = numberString.substring(0, length);
System.out.println(shortenedNumber); // 输出: 123
```
**JavaScript:**
```javascript
let numberString = "123456789";
let shortenedNumber = numberString.slice(0, 3);
console.log(shortenedNumber); // 输出: 123
```
相关问题
从键盘输入一个三阶方阵123456789再求其转置矩阵147258369并输出原矩阵和转置矩阵
要从键盘输入一个三阶方阵,可以按照以下步骤进行操作:
1. 首先,从键盘上输入九个数字,按照方阵的行优先顺序输入,即先输入第一行的三个数字,然后输入第二行的三个数字,最后输入第三行的三个数字。假设输入的数字为1、2、3、4、5、6、7、8、9。
2. 将这九个数字按照输入顺序填充到一个三阶方阵中,得到原矩阵。原矩阵如下所示:
1 2 3
4 5 6
7 8 9
3. 接下来,求原矩阵的转置矩阵。转置矩阵的定义是将原矩阵的行变为列,列变为行。对于这个例子,转置矩阵如下所示:
1 4 7
2 5 8
3 6 9
4. 最后,将原矩阵和转置矩阵输出。
原矩阵:
1 2 3
4 5 6
7 8 9
转置矩阵:
1 4 7
2 5 8
3 6 9
用简单的c++写一个代码,1 . 数据移位 有n(n<=10)个整数,使前面各数顺序向后移m (m<=n)个位置,最后m个数变成最前面的m个数,如图所示。编写函数shift(a,n,m)实现上述功能,在主函数中输入n个整数并输出调整后的n个数。 SAMPLE OUTPUT Input n(n<=10):10 Input 10 integers:123456789 10 Output the original:123456789 10 Input m(m<=10):4 Output the shifted:789 10123456
以下是C++的代码实现:
```c++
#include <iostream>
using namespace std;
void shift(int a[], int n, int m) {
int temp[m];
for (int i = n - m; i < n; i++) {
temp[i - (n - m)] = a[i]; // 保存最后m个数
}
for (int i = n - m - 1; i >= 0; i--) {
a[i + m] = a[i]; // 将前面n-m个数往后移m位
}
for (int i = 0; i < m; i++) {
a[i] = temp[i]; // 将保存的最后m个数放到最前面
}
}
int main() {
int n;
cout << "Input n(n<=10):";
cin >> n;
int a[n];
cout << "Input " << n << " integers:";
for (int i = 0; i < n; i++) {
cin >> a[i];
}
cout << "Output the original:";
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
int m;
cout << "Input m(m<=10):";
cin >> m;
shift(a, n, m);
cout << "Output the shifted:";
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
return 0;
}
```
输入样例:
```
Input n(n<=10):10
Input 10 integers:1 2 3 4 5 6 7 8 9 10
Input m(m<=10):4
```
输出样例:
```
Output the original:1 2 3 4 5 6 7 8 9 10
Output the shifted:7 8 9 10 1 2 3 4 5 6
```
阅读全文
相关推荐
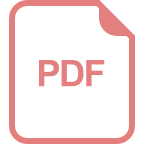
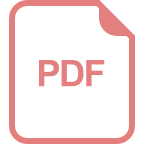
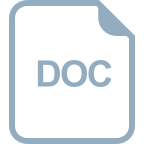














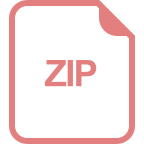