list如何链式添加元素
时间: 2023-09-13 12:11:13 浏览: 252
要实现链式添加元素,可以使用列表的 `append()` 方法。每次调用 `append()` 方法时,将新元素添加到列表的末尾。
以下是一个示例代码,演示了如何链式添加元素到列表中:
```python
my_list = []
my_list.append(1).append(2).append(3).append(4)
print(my_list) # 输出 [1, 2, 3, 4]
```
在这个示例中,我们创建了一个空列表 `my_list`,然后使用 `append()` 方法依次添加数值 1、2、3 和 4。每次调用 `append()` 方法后,返回的仍然是列表本身,因此可以继续调用 `append()` 方法进行链式操作。最后,我们打印输出了最终的列表内容。
请注意,链式添加元素只适用于 `append()` 方法,其他的列表方法(如 `extend()` 或索引赋值)不支持链式操作。
相关问题
建立一个包含3~5个数据元素的链式存储线性表
假设数据元素的类型为整数,可以建立如下的链式存储线性表:
```
struct Node {
int data;
Node* next;
};
Node* head = NULL;
Node* tail = NULL;
void addElement(int value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = NULL;
if (head == NULL) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
void printList() {
Node* current = head;
while (current != NULL) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
```
以上代码实现了向链表中添加数据元素和打印链表的功能。可以通过调用 `addElement` 函数向链表中添加数据,然后调用 `printList` 函数打印链表中的所有元素。例如,可以这样使用该链表:
```
addElement(1);
addElement(2);
addElement(3);
printList(); // 输出:1 2 3
```
以C结构体或C++的“类”代替“第2章中复杂数据类型”,实现“链式线性表”,编写下面6个接口函数:CreateList、ListPrint、GetElem、ListLength、ListInsert、ListDelete
### 回答1:
以下是使用C结构体实现链式线性表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int data;
struct ListNode* next;
};
struct ListNode* CreateList(int* arr, int n) {
struct ListNode* head = NULL;
struct ListNode* tail = NULL;
for (int i = 0; i < n; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->data = arr[i];
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
void ListPrint(struct ListNode* head) {
struct ListNode* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int GetElem(struct ListNode* head, int i) {
struct ListNode* p = head;
int j = 1;
while (p != NULL && j < i) {
p = p->next;
j++;
}
if (p == NULL || j > i) {
printf("GetElem: Index out of range\n");
return -1;
} else {
return p->data;
}
}
int ListLength(struct ListNode* head) {
struct ListNode* p = head;
int len = 0;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
void ListInsert(struct ListNode** head, int i, int x) {
struct ListNode* p = *head;
struct ListNode* prev = NULL;
int j = 1;
while (p != NULL && j < i) {
prev = p;
p = p->next;
j++;
}
if (j != i) {
printf("ListInsert: Index out of range\n");
return;
}
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->data = x;
node->next = p;
if (prev == NULL) {
*head = node;
} else {
prev->next = node;
}
}
void ListDelete(struct ListNode** head, int i) {
struct ListNode* p = *head;
struct ListNode* prev = NULL;
int j = 1;
while (p != NULL && j < i) {
prev = p;
p = p->next;
j++;
}
if (p == NULL || j != i) {
printf("ListDelete: Index out of range\n");
return;
}
if (prev == NULL) {
*head = p->next;
} else {
prev->next = p->next;
}
free(p);
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
struct ListNode* head = CreateList(arr, n);
ListPrint(head); // 输出:1 2 3 4 5
printf("ListLength: %d\n", ListLength(head)); // 输出:ListLength: 5
printf("GetElem(3): %d\n", GetElem(head, 3)); // 输出:GetElem(3): 3
ListInsert(&head, 2, 10);
ListPrint(head); // 输出:1 2 10 3 4 5
ListDelete(&head, 4);
ListPrint(head); // 输出:1 2 10 4 5
return 0;
}
```
说明:
- `struct ListNode` 结构体表示链表节点,包含一个 `data` 整型变量表示节点存储的数据,以及一个 `next` 指针变量表示下一个节点的地址。
- `CreateList` 函数用于创建链表,接受一个整型数组和数组长度作为参数,返回链表头节点的地址。在函数内部使用循环遍历数组,创建新节点并添加到链表尾部。
- `ListPrint` 函数用于输出链表中所有节点的数据。
- `GetElem` 函数用于获取链表中第 `i` 个节点的数据。在函数内部使用循环遍历链表,找到第 `i` 个节点并返回其数据。如果索引 `i` 超出了链表范围,则输出错误信息并返回 `-1`。
- `ListLength` 函数用于获取链表的长度。在函数内部使用循环遍历链表,计算节点个数并返回。
- `ListInsert` 函数用于在链表中第 `i` 个位置插入一个新节点,并将其数据设置为 `x`。在函数内部使用循环遍历链表,找到第 `i` 个节点的前一个节点,创建新节点并插入到链表中。如果索引 `i` 超出了链表范围,则输出错误信息。
- `ListDelete` 函数用于删除链表中第 `i` 个节点。在函数内部使用循环遍历链表,找到第 `i` 个节点的前一个节点,将其 `next` 指针跳过第 `i` 个节点并释放其内存。如果索引 `i` 超出了链表范围,则输出错误信息。注意需要使用指向指针的指针作为参数,以便在函数内部修改链表头节点的地址。
### 回答2:
构建链式线性表可以使用C结构体或C的“类”代替第2章中复杂数据类型。下面是6个接口函数的实现:
1. CreateList:创建链式线性表。
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* CreateList(int* arr, int length) {
Node* head = NULL;
Node* tail = NULL;
for (int i = 0; i < length; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
return head;
}
```
2. ListPrint:打印链式线性表。
```c
void ListPrint(Node* head) {
Node* p = head;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
3. GetElem:获取链式线性表指定位置处的元素值。
```c
int GetElem(Node* head, int position) {
Node* p = head;
int count = 0;
while (p && count < position) {
p = p->next;
count++;
}
if (p && count == position) {
return p->data;
} else {
return -1; // 返回-1表示获取元素失败
}
}
```
4. ListLength:获取链式线性表的长度。
```c
int ListLength(Node* head) {
Node* p = head;
int length = 0;
while (p) {
length++;
p = p->next;
}
return length;
}
```
5. ListInsert:在链式线性表的指定位置插入元素。
```c
void ListInsert(Node** head, int position, int data) {
if (position < 0) {
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
if (position == 0) {
newNode->next = *head;
*head = newNode;
} else {
Node* p = *head;
int count = 0;
while (p && count < position - 1) {
p = p->next;
count++;
}
if (p && count == position - 1) {
newNode->next = p->next;
p->next = newNode;
} else {
free(newNode);
return;
}
}
}
```
6. ListDelete:删除链式线性表的指定位置处的元素。
```c
void ListDelete(Node** head, int position) {
if (position < 0) {
return;
}
if (position == 0) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* p = *head;
int count = 0;
while (p && count < position - 1) {
p = p->next;
count++;
}
if (p && count == position - 1 && p->next) {
Node* temp = p->next;
p->next = p->next->next;
free(temp);
} else {
return;
}
}
}
```
### 回答3:
C结构体或C的“类”可以用来代替第2章中复杂数据类型,实现链式线性表。下面是编写的6个接口函数的实现:
1. CreateList:创建链式线性表。使用C结构体或C的“类”定义一个节点结构体,结构体中包含一个data字段和一个指向下一个节点的指针字段。通过动态内存分配,依次创建每个节点,并将节点链接起来形成链表。
2. ListPrint:打印链式线性表。遍历链表,依次输出每个节点的data字段的值。
3. GetElem:获取指定位置的元素。从链表的头节点开始,依次遍历指定位置之前的节点,直到找到指定位置的节点。返回该节点的data字段的值。
4. ListLength:获取链表的长度。遍历链表,每遍历一个节点,长度加1。
5. ListInsert:在指定位置插入元素。从链表的头节点开始,依次遍历指定位置之前的节点,找到该位置的前驱节点。新建一个节点,将要插入的元素赋值给节点的data字段,将新节点的指针字段指向前驱节点的下一个节点,将前驱节点的指针字段指向新节点。
6. ListDelete:删除指定位置的元素。从链表的头节点开始,依次遍历指定位置之前的节点,找到该位置的前驱节点。将前驱节点的指针字段指向要删除节点的下一个节点,释放要删除的节点的内存。
通过这6个接口函数的实现,可以实现链式线性表的基本操作,包括创建链表、打印链表、获取指定位置的元素、获取链表的长度、在指定位置插入元素、删除指定位置的元素。这些函数可以通过调用来完成对链式线性表的操作和管理。
阅读全文
相关推荐
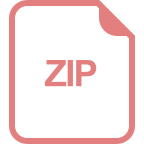
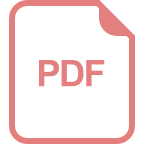
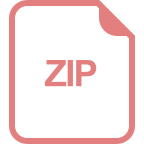
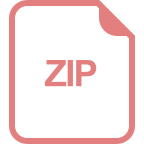
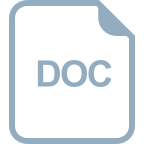
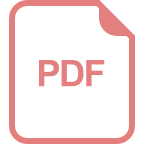
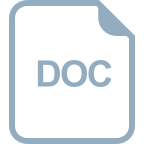









