输入四个整数,按由小到大的数据输出,至少写两个函数,另一个函数一定要在指针变量
时间: 2024-12-18 15:28:31 浏览: 3
当需要对输入的四个整数进行排序并输出时,可以设计两个函数,一个是直接排序并打印的主函数,另一个是辅助的比较函数,用于处理指针操作。这里假设我们使用C语言作为示例。
首先,创建一个函数`sort_and_print`,它接受四个整数作为参数,并通过内部排序算法(如冒泡排序)将它们排序:
```c
void sort_and_print(int a, int b, int c, int d) {
// 使用冒泡排序或其他排序方法
int temp;
if (a > b) {
temp = a;
a = b;
b = temp;
}
if (b > c) {
temp = b;
b = c;
c = temp;
}
if (c > d) {
temp = c;
c = d;
d = temp;
}
printf("Sorted numbers from smallest to largest: %d, %d, %d, %d\n", a, b, c, d);
}
```
然后,我们可以创建一个名为`compare_numbers`的辅助函数,该函数通常用于排序算法中的比较部分,但在这里我们将它作为参数传递给`sort_and_print`,以演示使用指针的概念:
```c
// 比较函数,接受指针作为参数
int compare(const void *a, const void *b) {
return (*(const int *)a - *(const int *)b);
}
// 主函数,通过指针调用compare_numbers
void sort_with_ptr(int *arr, int arr_size) {
qsort(arr, 4, sizeof(int), compare); // 使用qsort进行快速排序
sort_and_print(arr[0], arr[1], arr[2], arr[3]);
}
```
在这个例子中,`sort_with_ptr`函数接收一个整数数组的指针以及数组的大小,然后通过`qsort`函数利用`compare_numbers`进行排序,最后调用`sort_and_print`显示结果。
阅读全文
相关推荐



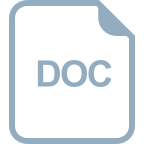






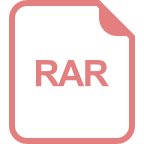


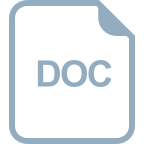
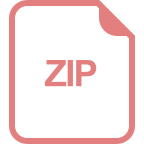
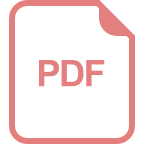