C++如何用一个变量承载函数,实现通过switch语句获取不同的函数并使用变量来承载
时间: 2024-12-22 19:14:30 浏览: 4
在C++中,可以使用`std::function`或`std::variant`以及模板来实现这样的功能。通常的做法是定义一个`std::function`,它可以存储不同类型的功能对象,然后在`switch`语句中动态地切换执行哪个函数。
例如:
```cpp
#include <functional>
#include <iostream>
// 定义一些要储存的函数指针类型
typedef void (func_type)(int);
void printHello(int x) {
std::cout << "Hello, the number is " << x << std::endl;
}
void printGoodbye(int x) {
std::cout << "Goodbye, the number is " << x << std::endl;
}
int main() {
// 创建函数指针容器
std::function<func_type> func;
// 存储函数
func = std::bind(&printHello, std::placeholders::_1);
switch (/* some condition */) {
case 0:
func(5); // 执行存储的printHello函数
break;
case 1:
func = std::bind(&printGoodbye, std::placeholders::_1);
func(7); // 这里会改变func指向的函数
break;
// 更多case...
}
return 0;
}
```
在这个例子中,`func`变量可以根据`switch`的条件改变存储的函数引用,并通过`func()`调用相应的函数。注意,`std::placeholders::_1`用于占位符匹配函数的参数。
阅读全文
相关推荐
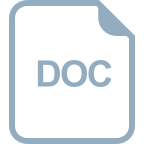
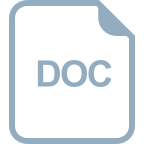
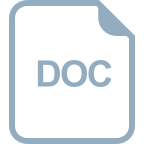
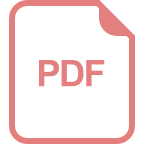
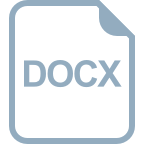
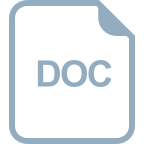
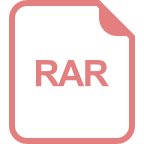
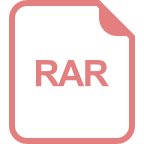
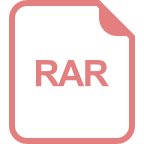
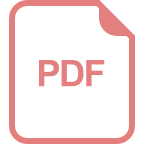
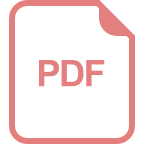
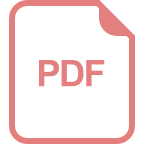
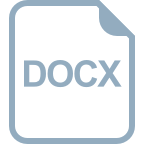
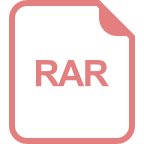
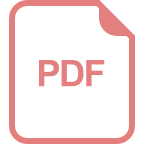
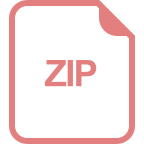
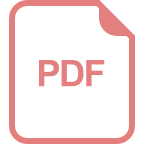
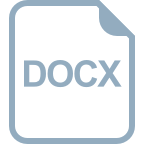
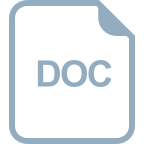