循环单链表基本操作c
时间: 2023-12-12 12:32:59 浏览: 90
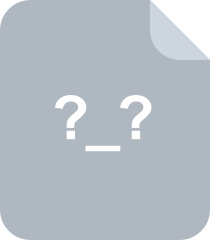
单链表的基本操作.c

循环单链表是一种链式存储结构,它的最后一个节点指向头节点,形成一个环。循环单链表的基本操作包括:创建、插入、删除、查找、遍历等。下面是循环单链表的基本操作C语言实现的示例代码:
1. 创建循环单链表
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createList() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = head;
return head;
}
```
2. 插入节点
```c
void insertNode(Node *head, int data, int pos) {
Node *p = head;
for (int i = 0; i < pos && p->next != head; i++) {
p = p->next;
}
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = p->next;
p->next = newNode;
}
```
3. 删除节点
```c
void deleteNode(Node *head, int pos) {
Node *p = head;
for (int i = 0; i < pos && p->next != head; i++) {
p = p->next;
}
Node *temp = p->next;
p->next = temp->next;
free(temp);
}
```
4. 查找节点
```c
int findNode(Node *head, int data) {
Node *p = head->next;
int pos = 0;
while (p != head) {
if (p->data == data) {
return pos;
}
p = p->next;
pos++;
}
return -1;
}
```
5. 遍历循环单链表
```c
void traverseList(Node *head) {
Node *p = head->next;
while (p != head) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
阅读全文
相关推荐


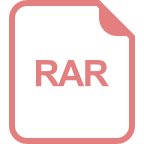
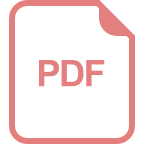
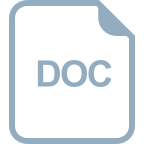
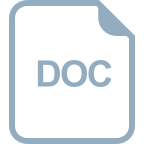
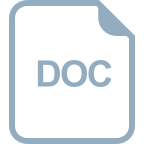
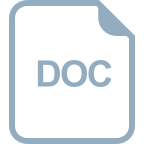





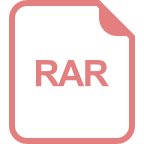
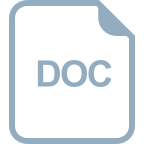
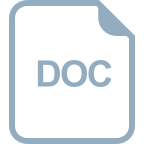