Elman 神经网络的代码
时间: 2024-04-07 21:25:52 浏览: 99
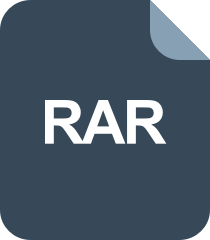
Elman 神经网络代码例子
Elman神经网络是一种循环神经网络(RNN),它具有记忆能力,可以处理序列数据。下面是一个简单的Elman神经网络的代码示例:
```python
import numpy as np
# 定义Elman神经网络类
class ElmanNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 初始化权重矩阵
self.Wxh = np.random.randn(hidden_size, input_size) * 0.01 # 输入层到隐藏层的权重矩阵
self.Whh = np.random.randn(hidden_size, hidden_size) * 0.01 # 隐藏层到隐藏层的权重矩阵
self.Why = np.random.randn(output_size, hidden_size) * 0.01 # 隐藏层到输出层的权重矩阵
# 初始化偏置向量
self.bh = np.zeros((hidden_size, 1)) # 隐藏层的偏置向量
self.by = np.zeros((output_size, 1)) # 输出层的偏置向量
def forward(self, inputs):
h = np.zeros((self.hidden_size, 1)) # 初始化隐藏层状态
self.hidden_states = [] # 存储每个时间步的隐藏层状态
for x in inputs:
h = np.tanh(np.dot(self.Wxh, x) + np.dot(self.Whh, h) + self.bh) # 更新隐藏层状态
self.hidden_states.append(h)
y = np.dot(self.Why, h) + self.by # 计算输出层的输出
return y, self.hidden_states
def backward(self, inputs, targets, learning_rate):
dWxh = np.zeros_like(self.Wxh)
dWhh = np.zeros_like(self.Whh)
dWhy = np.zeros_like(self.Why)
dbh = np.zeros_like(self.bh)
dby = np.zeros_like(self.by)
dh_next = np.zeros_like(self.hidden_states[0])
for t in reversed(range(len(inputs))):
dy = np.copy(self.outputs[t])
dy[targets[t]] -= 1 # 计算输出误差
dWhy += np.dot(dy, self.hidden_states[t].T) # 计算隐藏层到输出层权重的梯度
dby += dy # 计算输出层偏置的梯度
dh = np.dot(self.Why.T, dy) + dh_next # 计算隐藏层误差
dh_raw = (1 - self.hidden_states[t] ** 2) * dh # 计算隐藏层状态的梯度
dbh += dh_raw # 计算隐藏层偏置的梯度
dWxh += np.dot(dh_raw, inputs[t].T) # 计算输入层到隐藏层权重的梯度
dWhh += np.dot(dh_raw, self.hidden_states[t-1].T) # 计算隐藏层到隐藏层权重的梯度
dh_next = np.dot(self.Whh.T, dh_raw) # 传递到上一个时间步的隐藏层误差
# 更新权重和偏置
self.Wxh -= learning_rate * dWxh
self.Whh -= learning_rate * dWhh
self.Why -= learning_rate * dWhy
self.bh -= learning_rate * dbh
self.by -= learning_rate * dby
def train(self, inputs, targets, num_epochs, learning_rate):
for epoch in range(num_epochs):
outputs, hidden_states = self.forward(inputs)
loss = self.calculate_loss(outputs, targets)
self.backward(inputs, targets, learning_rate)
if (epoch+1) % 100 == 0:
print(f"Epoch: {epoch+1}, Loss: {loss}")
def calculate_loss(self, outputs, targets):
loss = 0
for t in range(len(targets)):
correct_word_predictions = outputs[t][targets[t]]
loss += -np.log(correct_word_predictions)
return loss
# 示例用法
input_size = 10
hidden_size = 20
output_size = 5
inputs = [np.random.randn(input_size, 1) for _ in range(5)]
targets = [np.random.randint(output_size) for _ in range(5)]
network = ElmanNetwork(input_size, hidden_size, output_size)
network.train(inputs, targets, num_epochs=1000, learning_rate=0.01)
```
这是一个简单的Elman神经网络的代码示例,其中包括前向传播、反向传播和训练过程。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐



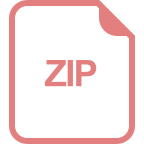
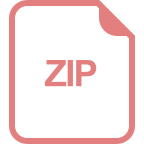
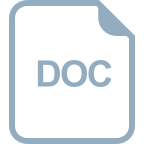
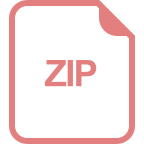
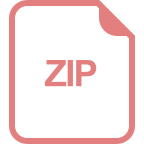
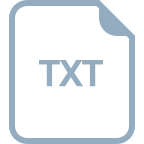
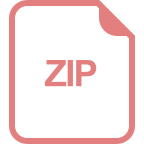
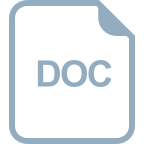
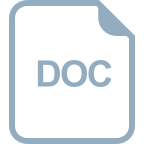
