pyton爬到的数据怎么保存到mysql数据库
时间: 2024-09-26 21:11:00 浏览: 26
Python爬取的数据通常会先存储在一个临时文件(如CSV、JSON或Pandas DataFrame),然后再导入到MySQL数据库。以下是基本步骤:
1. **数据处理**:
- 使用Python库(如`requests`抓取网页数据,`BeautifulSoup`解析HTML,`pandas`处理数据)将爬取的数据转换成DataFrame。
```python
import pandas as pd
data = requests.get('http://example.com').text
soup = BeautifulSoup(data, 'html.parser')
df = pd.DataFrame(soup.find_all('div', class_='data'))
```
2. **数据保存**:
- 将DataFrame保存到CSV或JSON文件,便于后续操作。
```python
df.to_csv('output.csv', index=False)
# 或者
df.to_json('output.json', orient='records')
```
3. **连接MySQL**:
- 使用`pymysql`库连接MySQL服务器。
```python
import pymysql
connection = pymysql.connect(host='localhost',
user='username',
password='password',
db='database_name',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor)
```
4. **数据导入**:
- 读取CSV或JSON文件,然后通过SQL查询插入到表中。
```python
with connection.cursor() as cursor:
if file_format == 'csv':
cursor.execute("LOAD DATA INFILE 'output.csv' INTO TABLE your_table")
elif file_format == 'json':
for row in open('output.json'):
data = json.loads(row)
query = "INSERT INTO your_table SET %s" % str(tuple(data.values()))
cursor.execute(query)
```
- 最后别忘了提交事务并关闭连接。
```python
connection.commit()
connection.close()
```
阅读全文
相关推荐
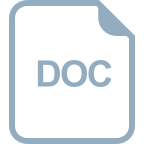
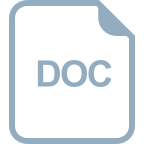
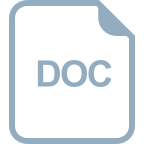
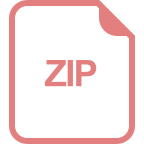
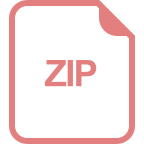
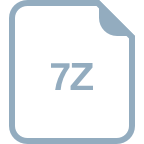
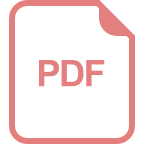
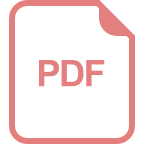
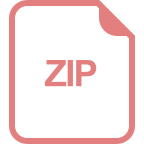
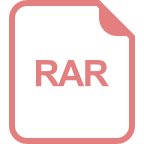







