设计网页,用JavaScript实现: 1)表单中,输入两个两位数的学号,其中有一个为你的学号最后2位 2)单击按钮,在页面显示学号和学号运算信息 3)计算结果显示红色
时间: 2024-10-09 17:13:15 浏览: 13
为了创建这样一个简单的网页,我们可以使用HTML、CSS和JavaScript。首先,我们来构建HTML结构:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>学号计算器</title>
<style>
.result {
color: red;
}
</style>
</head>
<body>
<h1>学号计算器</h1>
<form id="studentForm">
<label for="yourNumber">请输入您的最后两位学号:</label>
<input type="text" id="yourNumber" placeholder="XX" required><br>
<label for="anotherNumber">请输入另一个学号的后两位:</label>
<input type="text" id="anotherNumber" placeholder="XX" required><br>
<button onclick="calculate()">计算</button>
<div class="result" id="displayResult"></div>
</form>
<script src="script.js"></script>
</body>
</html>
```
接下来,我们在`script.js`文件中编写JavaScript逻辑:
```javascript
document.addEventListener('DOMContentLoaded', function() {
const yourNumberInput = document.getElementById('yourNumber');
const anotherNumberInput = document.getElementById('anotherNumber');
const displayResult = document.getElementById('displayResult');
function calculate() {
const yourNumber = parseInt(yourNumberInput.value);
const anotherNumber = parseInt(anotherNumberInput.value);
if (isNaN(yourNumber) || isNaN(anotherNumber)) {
displayResult.textContent = "请输入有效的数字!";
return;
}
// 假设这里有个简单的加法运算
let result = yourNumber + anotherNumber;
displayResult.textContent = `学号运算结果: ${result}`;
displayResult.classList.add('redIfNecessary'); // 添加红色样式(根据实际需求调整)
}
});
```
在这个例子中,当用户点击“计算”按钮时,我们会获取两个输入框的内容并尝试转换为整数。如果输入不是有效数字,我们会显示错误消息。然后进行简单的加法运算,并将结果显示在指定的元素上,同时添加一个CSS类使结果文本变为红色。
相关推荐
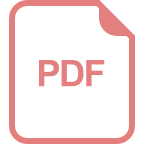
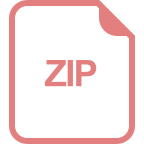
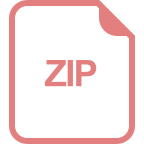














