void vTaskDelayUntil( TickType_t * const pxPreviousWakeTime, const TickType_t xTimeIncrement ) { TickType_t xTimeToWake; BaseType_t xAlreadyYielded, xShouldDelay = pdFALSE; configASSERT( pxPreviousWakeTime ); configASSERT( ( xTimeIncrement > 0U ) ); configASSERT( uxSchedulerSuspended == 0 ); vTaskSuspendAll(); { /* Minor optimisation. The tick count cannot change in this block. */ const TickType_t xConstTickCount = xTickCount; /* Generate the tick time at which the task wants to wake. */ xTimeToWake = *pxPreviousWakeTime + xTimeIncrement; if( xConstTickCount < *pxPreviousWakeTime ) { /* The tick count has overflowed since this function was lasted called. In this case the only time we should ever actually delay is if the wake time has also overflowed, and the wake time is greater than the tick time. When this is the case it is as if neither time had overflowed. */ if( ( xTimeToWake < *pxPreviousWakeTime ) && ( xTimeToWake > xConstTickCount ) ) { xShouldDelay = pdTRUE; } else { mtCOVERAGE_TEST_MARKER(); } } else { /* The tick time has not overflowed. In this case we will delay if either the wake time has overflowed, and/or the tick time is less than the wake time. */ if( ( xTimeToWake < *pxPreviousWakeTime ) || ( xTimeToWake > xConstTickCount ) ) { xShouldDelay = pdTRUE; } else { mtCOVERAGE_TEST_MARKER(); } } /* Update the wake time ready for the next call. */ *pxPreviousWakeTime = xTimeToWake; if( xShouldDelay != pdFALSE ) { traceTASK_DELAY_UNTIL( xTimeToWake ); /* prvAddCurrentTaskToDelayedList() needs the block time, not the time to wake, so subtract the current tick count. */ prvAddCurrentTaskToDelayedList( xTimeToWake - xConstTickCount, pdFALSE ); } else { mtCOVERAGE_TEST_MARKER(); } } xAlreadyYielded = xTaskResumeAll(); /* Force a reschedule if xTaskResumeAll has not already done so, we may have put ourselves to sleep. */ if( xAlreadyYielded == pdFALSE ) { portYIELD_WITHIN_API(); } else { mtCOVERAGE_TEST_MARKER(); } }
时间: 2024-04-18 20:24:39 浏览: 24
这是 FreeRTOS 中的 `vTaskDelayUntil()` 函数的实现代码。
该函数用于使任务进入阻塞状态,直到指定的时间点(`pxPreviousWakeTime` + `xTimeIncrement`)到达或超过当前系统的 tick 计数器的值。
具体实现逻辑如下:
1. 首先,函数会进行一系列的断言检查,以确保参数的有效性和调用环境的正确性。
2. 然后,函数会挂起调度器,禁止任务切换。
3. 在挂起调度器的情况下,函数会获取当前系统的 tick 计数器的值,并计算出任务希望唤醒的时间点 `xTimeToWake`。
4. 如果当前系统的 tick 计数器发生了溢出,那么只有在唤醒时间也发生了溢出且唤醒时间大于 tick 计数器时,才会真正进行延迟操作。否则,不进行延迟操作。
5. 更新 `pxPreviousWakeTime` 的值为下一次调用时的唤醒时间。
6. 如果需要进行延迟操作,则将当前任务添加到延迟列表中。
7. 恢复调度器的状态,并根据是否已经执行了任务切换来决定是否强制进行一次任务切换。
总之,`vTaskDelayUntil()` 函数根据指定的时间点来决定是否阻塞任务,并在适当的时机进行任务切换,以实现精确的定时功能。
相关问题
char*转const uint8_t *const*
要将`char*`转换为`const uint8_t *const*`类型,你需要进行多级的指针转换。下面是一个示例:
```cpp
char* charPtr = "example";
const uint8_t* const* uintPtr = reinterpret_cast<const uint8_t* const*>(&charPtr);
```
在上述示例中,我们首先使用`reinterpret_cast`将`char*`转换为`const uint8_t*`类型的指针。然后,我们再次使用`reinterpret_cast`将该指针转换为`const uint8_t* const*`类型的指针。
请注意,这种多级指针转换也是非常危险的,因为它依赖于底层的指针表示方式和内存布局。确保你对这种转换有充分的理解,并明确了解风险和潜在的问题。
请注意,这只是一种可能的解决方案,具体取决于你的代码和需求。如果你能提供更多的上下文或代码片段,我可以给出更具体的建议。
char*转const uint8_t *const
要将`char*`转换为`const uint8_t * const`类型,你可以使用类型转换来实现。下面是一个示例:
```cpp
char* charPtr = "example";
const uint8_t* const uintPtr = reinterpret_cast<const uint8_t* const>(charPtr);
```
上述代码中,`reinterpret_cast`用于进行类型转换,将`char*`类型的指针转换为`const uint8_t* const`类型的指针。注意,这个转换是非常危险的,因为它依赖于底层的指针表示方式和内存布局。确保你对这种转换有充分的理解,并明确了解风险和潜在的问题。
请注意,这只是一种可能的解决方案,具体取决于你的代码和需求。如果你能提供更多的上下文或代码片段,我可以给出更具体的建议。
相关推荐
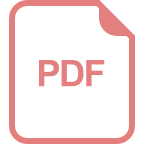
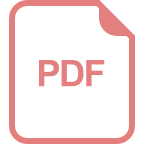
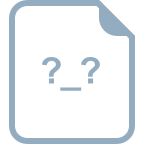












