现在有一个包含n个整数的数组num, 请输出任意一个至少出现了三次的数字,如果不存在任何一个数字出现了三次及以上,则输出 -1,用c语言。
时间: 2024-10-14 09:07:55 浏览: 7
在C语言中,你可以通过哈希表(使用数组或者动态分配内存的结构如`unordered_map`或`std::map`)来解决这个问题。以下是一个简单的示例,使用数组作为哈希表的实现:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_NUM 1000 // 根据实际情况调整最大值
int findThreeTimes(int arr[], int n) {
bool hash[MAX_NUM] = {false}; // 哈希表,初始化所有元素为false
for (int i = 0; i < n; i++) {
if (hash[arr[i]] == true) {
return arr[i]; // 如果找到重复的,返回该数字
}
hash[arr[i]] = true; // 标记当前数字已存在
}
// 没有找到至少出现三次的数字,返回-1
return -1;
}
int main() {
int num[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 9, 9, 10};
int n = sizeof(num) / sizeof(num[0]);
int result = findThreeTimes(num, n);
printf("The number that appears at least three times is: %d\n", result);
return 0;
}
```
这个程序首先遍历数组,对于每个元素,检查它是否已经在哈希表中。如果在,说明找到了重复的数字,直接返回。如果没有,则标记它为已存在。如果遍历完整个数组都没有找到重复的,那么返回-1。
阅读全文
相关推荐
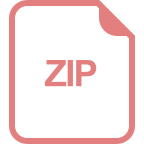
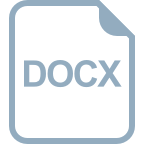
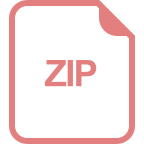



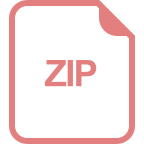
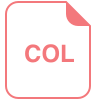
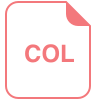
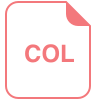
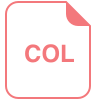
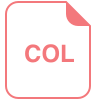
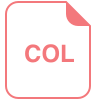
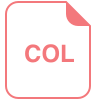
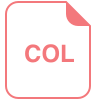
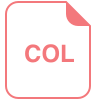
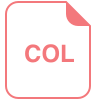